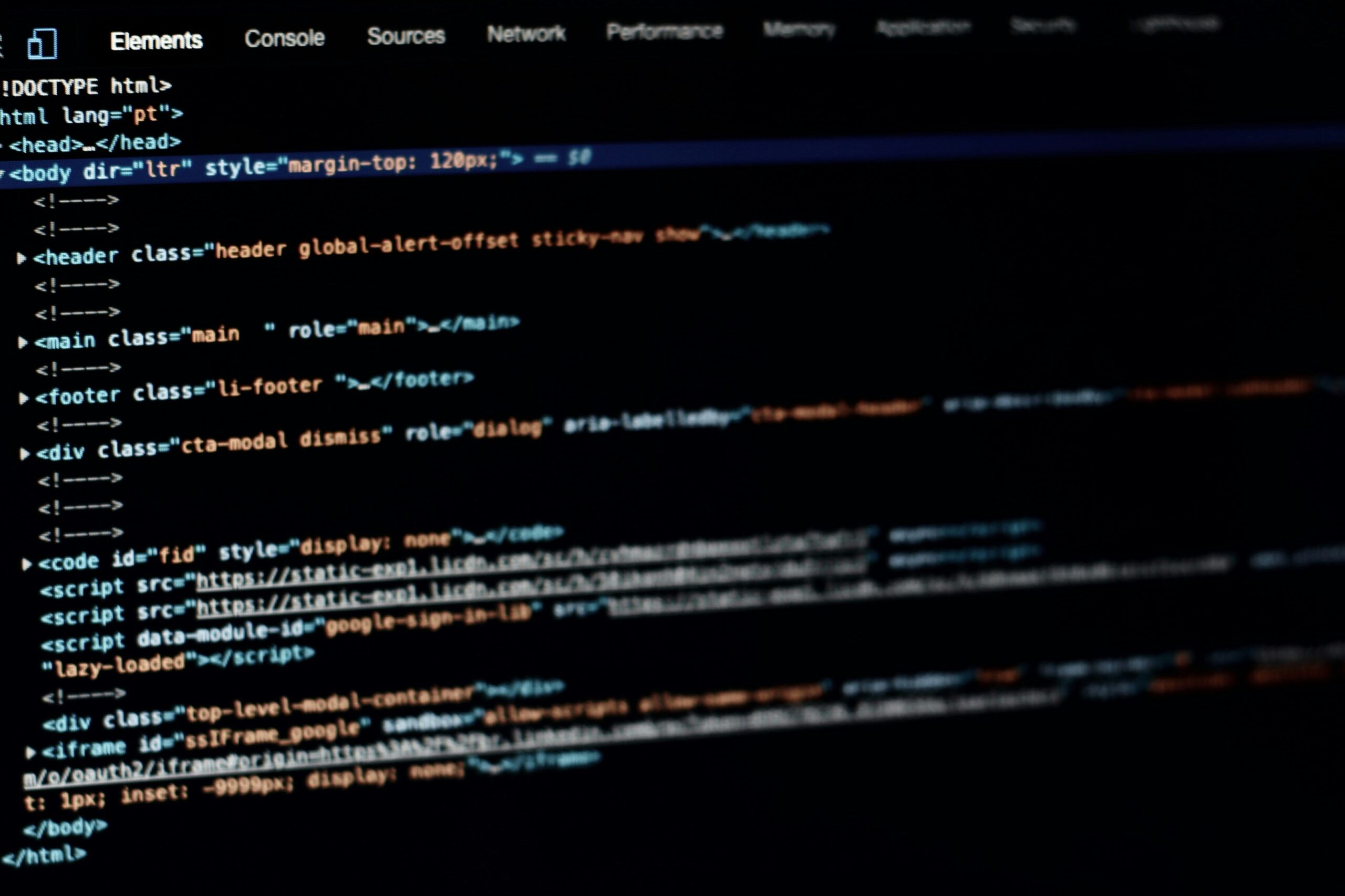
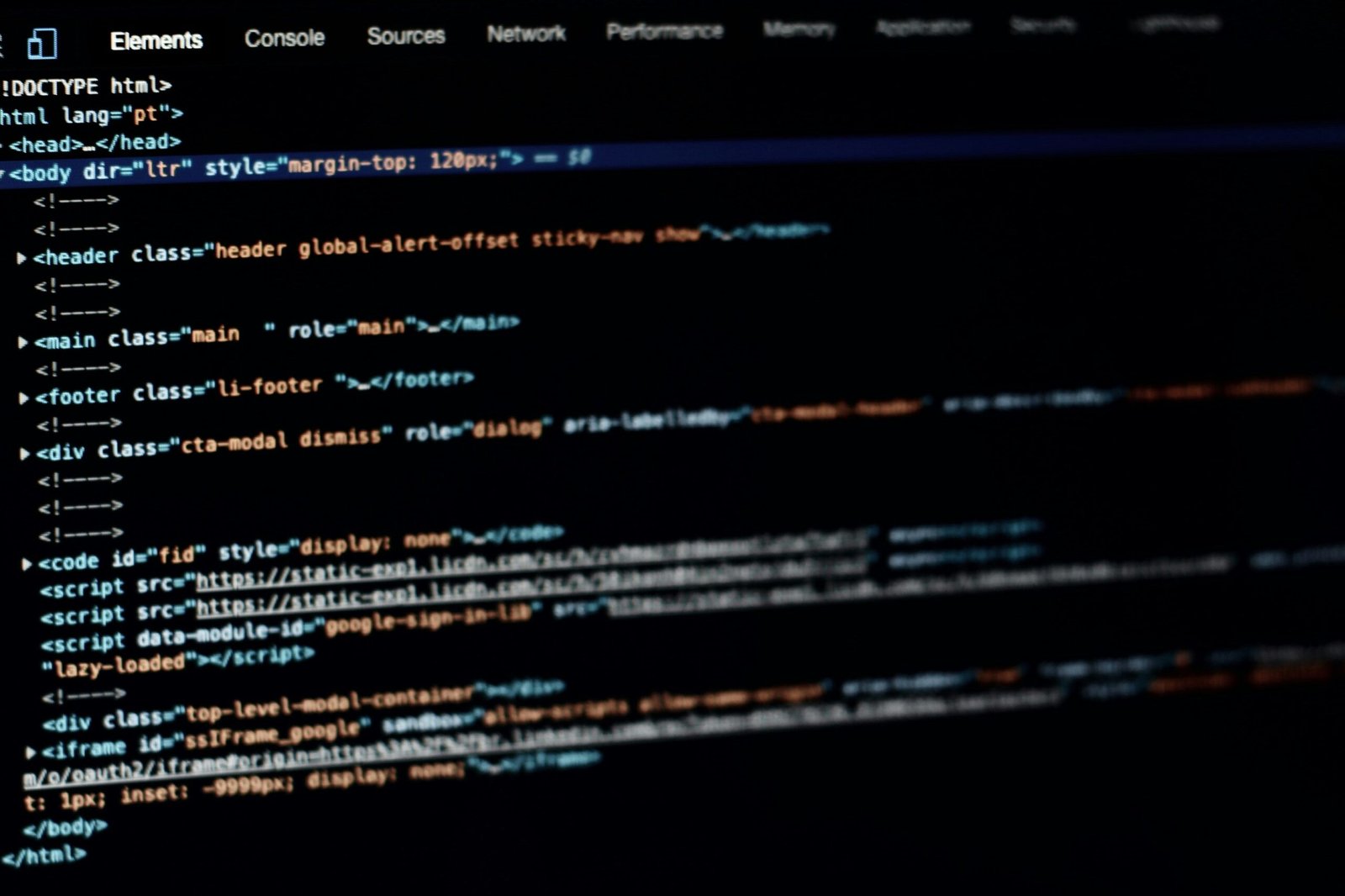
Introduction to F#
F# is a powerful, functional-first programming language developed by Microsoft Research, known for its efficiency in handling complex computational tasks. As part of the .NET family, F# blends elements of functional programming with the .NET ecosystem, enabling developers to write succinct and robust code. It emerged in the early 2000s, drawing inspiration from the ML programming language family, particularly OCaml, and was officially released in 2005. Today, F# is a well-established language, supporting a wide range of applications from data analysis to web development.
The uniqueness of F# lies in its seamless integration with both functional and object-oriented programming paradigms. Functional programming encourages immutability, first-class functions, and the use of expressions rather than statements. These principles inherently contribute to fewer bugs and more predictable code, making F# an appealing choice for tasks requiring high reliability and maintainability.
One of the primary objectives behind F# is to make the powerful concepts of functional programming accessible and more actionable within the .NET environment. This cross-paradigm flexibility allows developers to leverage the efficiencies of functional programming while capitalizing on the robustness and extensive libraries of the .NET framework. This synergy simplifies the development of highly scalable and performant applications.
Key features that set F# apart include pattern matching, type inference, and asynchronous programming capabilities. Pattern matching allows for concise and expressive code by enabling developers to easily deconstruct data structures. Type inference minimizes the need for explicit type annotations, promoting cleaner and more readable code. Additionally, F# offers advanced support for asynchronous workflows, which is particularly beneficial in the development of high-performance, responsive applications.
F# has carved a niche for itself in the realms of scientific computing, financial modeling, and machine learning, thanks to its clarity and precision. The language’s ability to handle complex data transformations effortlessly makes it a favorite among data scientists and financial analysts. By championing a functional-first approach, F# continues to push the boundaries of what developers can achieve in the .NET ecosystem and beyond.
Core Concepts of F#
F# is a functional-first programming language that emphasizes immutability and concise syntax, making it distinctly different from many traditional languages. At its core, functional programming promotes the use of immutable data structures and pure functions. In F#, this is achieved through several key principles and features.
First and foremost, immutability in F# means that once a data structure is created, it cannot be changed. This practice leads to more predictable and maintainable code. To define a variable, F# uses let bindings. For example, let x = 5
creates an immutable binding of x
to the value 5.
A fundamental principle of F# is the concept of first-class functions, where functions are treated as first-class citizens. This implies that functions can be passed as arguments, returned from other functions, and assigned to variables. First-class functions enable higher-order functions, which can take other functions as parameters or return them, promoting modularity and reusability.
Pattern matching is another powerful feature of F#. This mechanism allows for the decomposition of data structures and the application of expressions based on the structure’s shape. Pattern matching is used extensively in handling lists, tuples, and various complex data types. For example, a match expression can evaluate different cases for list processing: match myList with | [] -> "Empty" | h::t -> "Head and Tail"
.
F# also excels in asynchronous programming, supporting non-blocking operations neatly. The async workflow in F# helps developers write clear and concise asynchronous code. By using async constructs, tasks such as I/O operations can be executed without blocking the main thread, leading to performance improvements in concurrent applications.
In summary, the core concepts of F#—including functional programming paradigms, immutability, first-class functions, and asynchronous workflows—combined with its elegant syntax and powerful constructs like pattern matching, make it an expressive language well-suited for modern software development.
Benefits and Use Cases
F# is renowned for several compelling benefits that make it an attractive choice for developers. One of the foremost advantages is its ability to produce concise code. The succinct syntax of F# reduces the amount of boilerplate code required, thereby enhancing readability and speeding up the coding process. This feature is particularly advantageous in projects where quick iterations and clarity are essential.
Another significant advantage of F# is its ease of maintenance. The language’s strong type inference system helps in the early detection of errors, facilitating a more efficient debugging process. Moreover, F# belongs to the ML family of programming languages known for their immutable data structures, which intrinsically promote code stability and reduce side effects. These characteristics contribute to robust and maintainable codebases, making F# a preferred choice for long-term projects.
F# provides robust support for concurrency and parallelism, crucial for high-performance applications. Alongside its powerful asynchronous workflows, F#’s lightweight threading model makes it possible to achieve efficient parallel processing. These features are indispensable for applications that demand high throughput and performance, such as real-time financial systems and computational science platforms.
The applications of F# extend to various industries, showcasing its versatility and effectiveness. In the realm of data processing, F# is frequently employed due to its efficient handling of large datasets and robust mathematical computations. Its functional programming paradigm excels in transforming and analyzing complex data structures with ease.
Financial modeling is another domain where F# shines. The language’s ability to quickly and accurately perform numerical analyses is beneficial for crafting financial models and simulations. Many financial institutions leverage F# to develop trading algorithms, risk assessment tools, and quantitative analysis systems.
In scientific computing, F#’s extensive library support and efficient computational capabilities make it an ideal choice for researchers and scientists. The language’s robust infrastructure facilitates the development of complex simulations, statistical models, and data analysis tools, thereby accelerating the pace of scientific discoveries.
Setting Up the F# Development Environment
Setting up the F# development environment is a crucial step for any developer looking to leverage the strengths of this functional-first programming language. A properly configured environment not only ensures smooth coding experiences but also enhances productivity. This section outlines the required software and tools and provides step-by-step instructions for setting up your F# development environment.
To start with, you need to install the .NET SDK, which provides the necessary runtime and libraries for running F# applications. The .NET SDK can be downloaded from the official .NET website. During installation, follow the prompts to ensure that all components are correctly installed.
Next, you need the F# compiler, which is typically included in the .NET SDK. However, it’s advisable to verify its inclusion by running the command dotnet --list-sdks
in your terminal. If F# is listed among the SDKs, you are good to go. Should it not be included, a separate installation of the F# compiler from the F# Software Foundation website is necessary.
For a robust development environment, using an Integrated Development Environment (IDE) like Visual Studio or an advanced code editor like Visual Studio Code is highly recommended. Visual Studio offers comprehensive tools and features that are particularly useful for larger projects. It can be downloaded from the official Visual Studio website, with installation options available to include F# support specifically. Visual Studio Code, a lightweight alternative, provides F# language support through extensions, namely “Ionide-fsharp.” This extension can be easily added via the Visual Studio Code marketplace.
Once the necessary installations are complete, configuring your environment properly can significantly enhance productivity. Customize your IDE or code editor with plugins and extensions that complement F#. Features like auto-completion, syntax highlighting, and integrated debugging are particularly beneficial. Adjusting settings to suit your workflow, such as keyboard shortcuts and interface layout, can also streamline your development process.
In conclusion, setting up an F# development environment primarily involves installing the .NET SDK, F# compiler, and an appropriate IDE or code editor. Proper configuration tailored to your working style can lead to a more productive and enjoyable development experience.
Basic Programming with F#
F# is known for its succinct and expressive syntax, which makes it an excellent choice for both beginners and experienced developers. To begin with, one must understand the foundational elements of F# programming, including writing simple programs, working with variables, control structures, and functions.
In F#, programs are written in script files that typically have a `.fsx` extension. Let’s start with a simple program to print “Hello, World!” to the console.
```fsharpprintfn "Hello, World!"```
This succinct code snippet illustrates the use of the `printfn` function, which is employed to output text to the console. The `%` sign within the `printfn` function allows more complex formatting but isn’t necessary for this simple example.
Variables in F# are defined using the `let` keyword. For instance:
```fsharplet x = 42let message = "The answer to life, the universe, and everything"```
The immutability of values assigned to variables is a core aspect of F#’s design philosophy. However, when mutable variables are required, one can use the `mutable` keyword:
```fsharplet mutable counter = 0counter <- counter + 1```
Control structures in F# include conditional statements, loops, and pattern matching. A simple `if`-`else` conditional statement looks like this:
```fsharplet number = 10if number % 2 = 0 thenprintfn "Even"elseprintfn "Odd"```
For iteration, the `for` loop and `while` loop are commonly used. Here’s an example of a `for` loop:
```fsharpfor i in 1 .. 5 doprintfn "Value: %d" i```
Pattern matching is another powerful feature of F#, allowing more complex control flows with concise syntax:
```fsharplet classifyNumber x =match x with| 0 -> "Zero"| 1 -> "One"| _ -> "Other"```
Functions form the backbone of F# programming, encapsulating reusable code blocks. A basic function is defined as follows:
```fsharplet add a b = a + blet result = add 3 5printfn "Sum: %d" result```
F# functions are often concise and integrate seamlessly with the language’s functional programming paradigm. These core concepts provide a robust foundation for developing more sophisticated applications using F#.
Advanced Features and Techniques
F# offers a rich set of advanced features and techniques that enable developers to create more robust and efficient applications. Among these, type providers, computation expressions, and custom operators stand out due to their powerful capabilities and practical applications in various scenarios.
Type providers are a compelling feature in F# that provide a way to access data in a strongly typed manner. They make it easier to work with external data sources, such as databases, web services, or even file formats like XML and JSON, by automatically generating types based on the schema of the data. This not only reduces the amount of boilerplate code but also enhances the reliability of your code by ensuring type safety. For instance, a type provider for an SQL database can generate types for tables and fields, allowing developers to write queries in F# with full IntelliSense support and compile-time checking.
Computation expressions in F# offer a powerful way to work with complex computations, such as asynchronous workflows, sequences, or even custom computation models. They are a syntactic convenience that allows developers to define computations in a more readable and maintainable way using a DSL (Domain Specific Language). An example is the use of asynchronous workflows to handle non-blocking IO operations efficiently. By leveraging computation expressions, developers can write concise and clear code for tasks that would otherwise require more extensive boilerplate and state management.
Custom operators in F# provide the flexibility to define new operators or overload existing ones, enabling developers to create more expressive code. This feature is especially useful in domain-specific applications where custom infix functions can significantly improve readability and maintainability. For instance, in mathematical or financial applications, defining custom operators for specific calculations can make the code resemble the domain language closely, thus reducing the cognitive load for domain experts who need to read and understand the codebase.
Overall, these advanced features and techniques make F# a versatile language well-suited for a range of applications, from data-intensive tasks to highly parallel computations. By integrating type providers, computation expressions, and custom operators into your development practices, you can leverage the full potential of F# and build more expressive, efficient, and maintainable software solutions.
F# in the Ecosystem
F# holds a unique position in the broader software development ecosystem. It operates within the .NET framework, making it relatively easy to integrate with other .NET languages such as C#. This interoperability is a significant advantage for organizations that already utilize C# for application development. The compatibility between F# and C# ensures that developers can leverage libraries and tools from both languages, facilitating a seamless and efficient development process.
Comparatively, F# shares some syntactic and functional paradigms with Python, making it appealing to developers accustomed to Python’s expressive and concise coding style. However, where F# distinguishes itself is in its strong typing and functional programming capabilities, which can reduce runtime errors and improve the predictability of code. These features are particularly beneficial in complex systems where reliability is paramount.
The F# community, though smaller than those of more mainstream languages, is notably active and supportive. This tight-knit community fosters an environment where developers can share projects, collaborate on open-source initiatives, and contribute to the language’s evolution. Numerous open-source projects, such as the F# Compiler Service and Ionide — an F# extension for Visual Studio Code — exemplify the community’s contributions to making F# more accessible and powerful.
For those new to F#, a rich array of resources is available to facilitate learning and mastery. Online platforms such as fsharp.org offer an abundance of tutorials, documentation, and best practices guides. Additionally, various community-led initiatives, including local meetups and conferences, provide opportunities for developers to network and deepen their understanding of the language.
In summary, F# carves out a distinctive niche in the programming landscape. Its interoperability with C#, shared features with Python, and a vibrant community make it a compelling choice for developers seeking a robust and versatile programming language. The growing collection of resources ensures that newcomers have ample support as they embark on their journey with F#.
Real-World Examples and Case Studies
In recent years, F# has emerged as a potent tool in various domains, including finance, data science, and web development. A notable example is the use of F# at Jet.com, an eCommerce platform, where its functional programming features have significantly streamlined the company’s backend systems. The integration of F# in their system helped to simplify complex pricing algorithms, enhancing computational efficiency and reducing codebase maintenance. Furthermore, Jet.com leveraged F# to facilitate seamless integration with other .NET languages, thus ensuring robust and scalable software architecture.
Another compelling case study involves the use of F# in the financial services industry, particularly at Credit Suisse. This global investment bank utilized F# to build a domain-specific language that improves risk modeling and financial analysis. The primary challenge faced was the need for a resilient yet flexible system capable of handling high-frequency trading data. F# provided robust type-safety and succinct syntax that enabled rapid development cycles while minimizing the margin for error. This level of precision and reliability significantly contributed to the bank’s risk mitigation strategies.
In the realm of data science, F# has proven its mettle at Microsoft Research. Here, it was employed in the development of Infer.NET, a machine learning framework. The project aimed to perform probabilistic programming to support complex analytical models. F#’s functional programming paradigms offered advanced recursion and higher-order functions, which were instrumental in efficiently calculating probabilistic inferences. This facilitated groundbreaking research and accelerated advancements in predictive analytics.
Across these varied spectrum of industries, the adoption of F# has demonstrated its ability to tackle industry-specific challenges. Whether manipulating large datasets or simplifying intricate algorithms, the language’s functional strengths have proven valuable. The aforementioned case studies underscore how F# not only resolves current operational hurdles but also provides scalability for future growth.