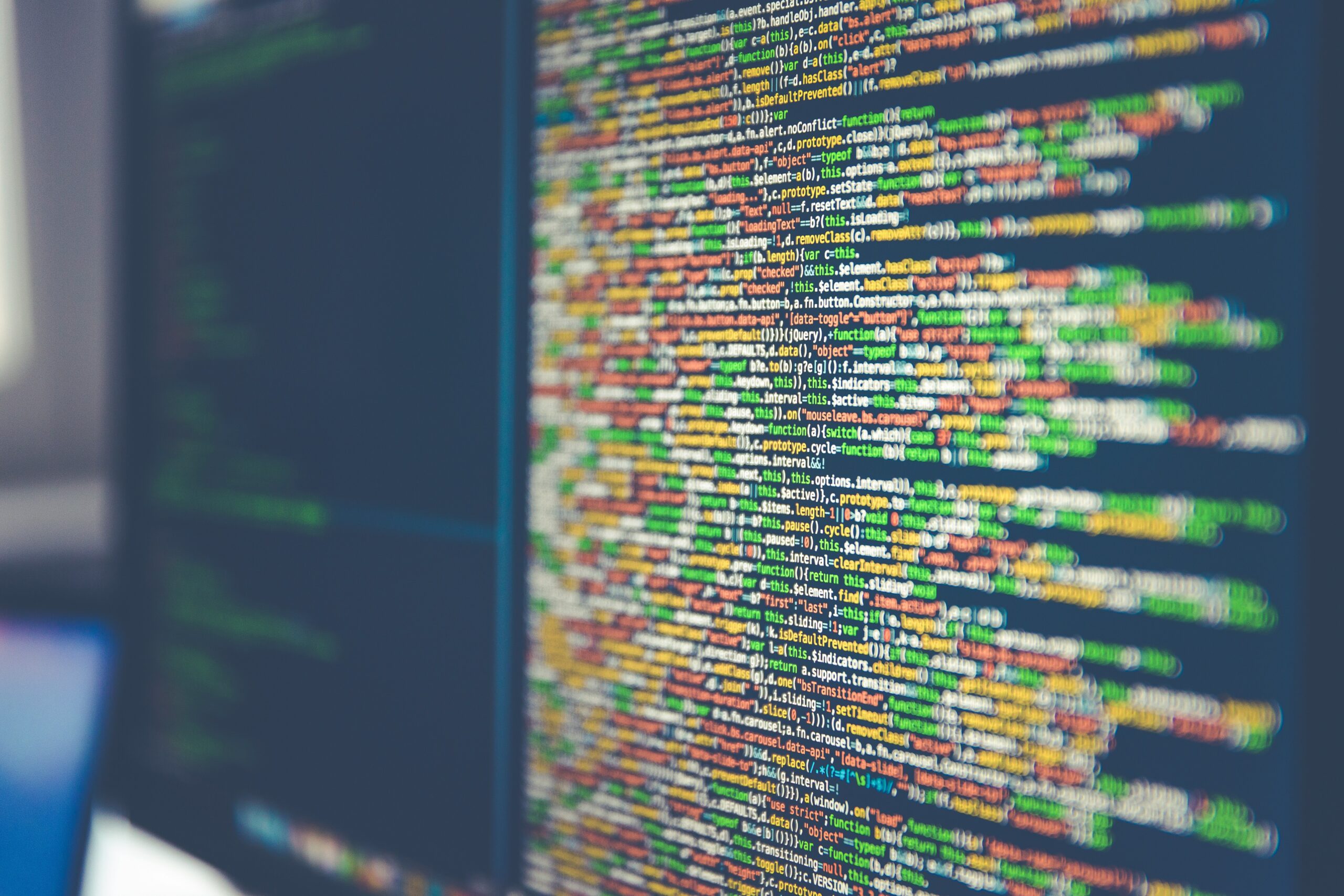
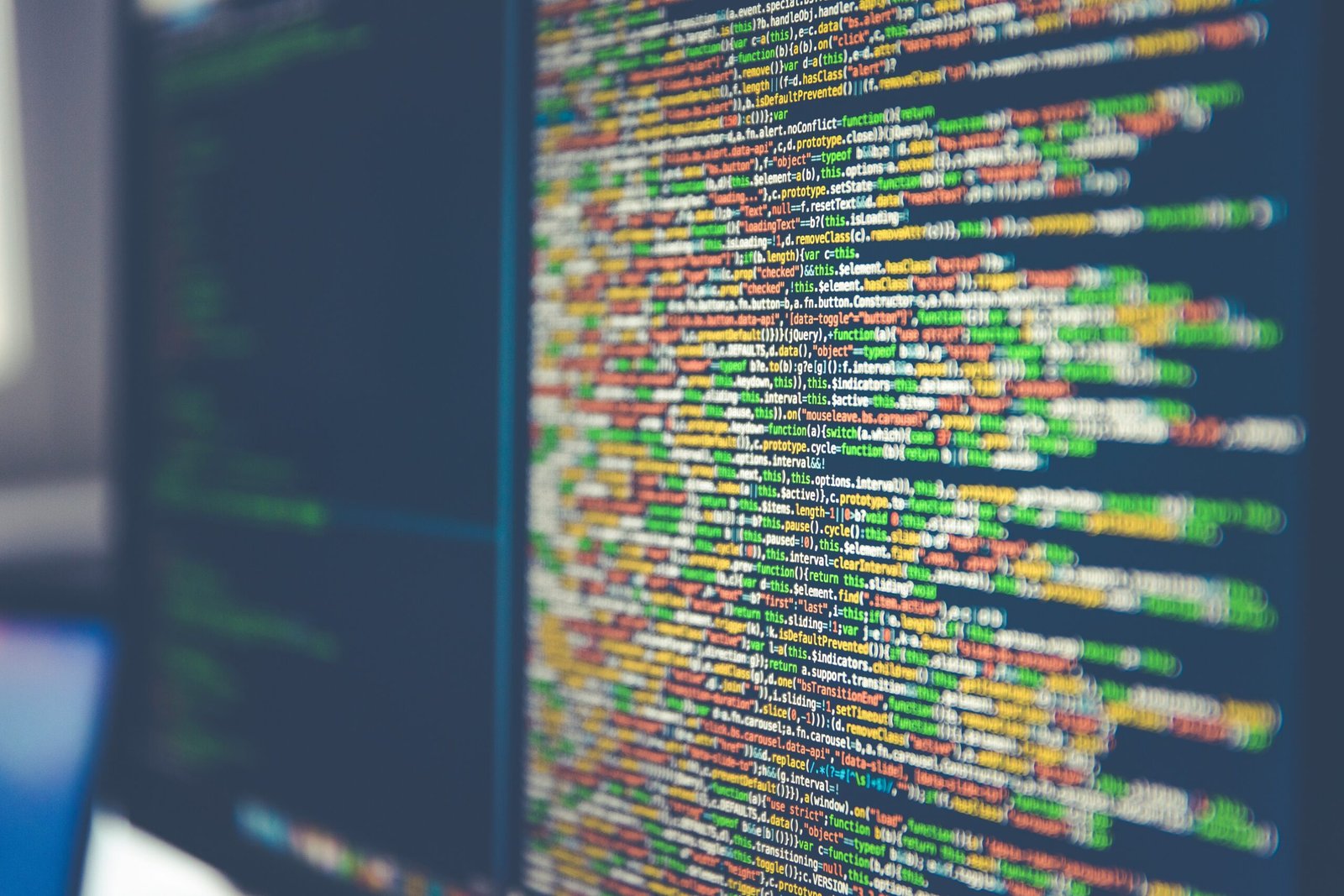
Introduction to Object-Oriented Programming
Object-Oriented Programming (OOP) has shaped the landscape of modern software development since its inception. The concept finds its roots in the late 1960s, with languages like Simula, which introduced the idea of “objects” as a way to model real-world entities. As the model evolved, OOP gained widespread adoption in the 1980s thanks to languages such as Smalltalk and C++, and later with Java in the 1990s. Today, OOP is a foundational paradigm in software engineering, influencing a wide array of programming languages, including Python, C#, and JavaScript.
At its core, OOP is based on the principle of modeling software systems as collections of objects. Each object is an encapsulation of data (attributes) and behaviors (methods). This stands in contrast to older procedural programming paradigms, which focus on sequences of instructions and the manipulation of data through function calls. By integrating data and behavior within objects, OOP facilitates more modular, scalable, and maintainable code.
The shift from procedural to object-oriented paradigms was driven by a growing need for complexity management in software projects. As systems became larger and more intricate, procedural codebases often turned cumbersome and unwieldy. OOP addressed these issues by promoting modularity through encapsulation, inheritance, and polymorphism—thereby enabling developers to break down complex systems into more manageable, reusable components. These OOP concepts have proven instrumental in enhancing software design principles, leading to more efficient development processes.
Moreover, OOP’s model aligns closely with real-world logic, making it intuitive for developing systems that map directly to real-world entities and interactions. This alignment not only simplifies the design process but also enhances collaboration among development teams, as the code structure can be easily understood and communicated.
Through its evolution, OOP has established itself as a cornerstone of contemporary software engineering, shaping the way developers think about and construct software solutions. Its principles and practices continue to drive innovation and efficiency in the field, making it an indispensable paradigm for modern programming.
Core Principles of Object-Oriented Programming
Object-Oriented Programming (OOP) is anchored by four core principles: Encapsulation, Abstraction, Inheritance, and Polymorphism. Understanding these principles is crucial for creating modular, reusable, and maintainable code.
Encapsulation refers to bundling the data (attributes) and the methods (functions) that operate on the data into a single unit, often called an object. It restricts direct access to some of an object’s components, which is a means of preventing unintended interference and misuse of the data. For instance, consider a `Person` class with private attributes like `name` and `age` and public methods like `getName` and `setName`. This encapsulation safeguards the internal state of the object.
Abstraction simplifies complex systems by modeling classes appropriate to the problem, and leaves out the unnecessary details. This principle allows a programmer to focus on interactions at a high level, without needing to manage the intricate details. For example, in a banking system, an `Account` class with methods `deposit` and `withdraw` abstracts the more complex internal operations of updating the account balance. Abstraction helps in managing large systems by decomposing the system into smaller, more manageable pieces.
Inheritance is a mechanism wherein a new class inherits properties and behavior (methods) from an existing class. This principle allows for the creation of a new class with enhanced functionality based on an existing class. For instance, a `Vehicle` class may have subclasses like `Car` and `Truck`, which inherit the common properties from `Vehicle` but also have their own unique features. Inheritance promotes code reusability and establishes a natural hierarchy.
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It is particularly useful when you want to call the same method on different objects that behave differently. For example, a `Shape` class may have subclasses such as `Circle` and `Square`, each implementing a `draw` method. Through polymorphism, a developer can call `draw` on a `Shape` reference, and the correct method corresponding to the actual object’s type will be executed. Polymorphism increases the flexibility and maintainability of the code.
Encapsulation, Abstraction, Inheritance, and Polymorphism collectively enable developers to design and implement more efficient, manageable, and scalable software systems, making OOP a powerful paradigm in modern software development.
Classes and Objects in OOP
In the realm of Object-Oriented Programming (OOP), classes and objects form the fundamental building blocks. A class can be regarded as a blueprint or prototype from which objects are created. It encapsulates data for the object and methods to manipulate that data. In simpler terms, a class defines the characteristics and behaviors that the objects created from the class will have.
Instances of classes are known as objects. An object, then, is an instance of a class. When a class is created, its properties and methods are not directly usable; only when an object is instantiated from the class can these attributes and functionalities be utilized. Consider a class as a cookie cutter and an object as the cookie itself – the cutter defines the shape, but the actual cookie is the edible product.
For better comprehension, let’s look at examples in different programming languages:
Python Example
“`pythonclass Car:def __init__(self, brand, model, year):self.brand = brandself.model = modelself.year = yeardef display_info(self):return f”{self.year} {self.brand} {self.model}”my_car = Car(‘Toyota’, ‘Corolla’, 2020)print(my_car.display_info())“`
Java Example
“`javapublic class Car {String brand;String model;int year;public Car(String brand, String model, int year) {this.brand = brand;this.model = model;this.year = year;}public String displayInfo() {return year + ” ” + brand + ” ” + model;}public static void main(String[] args) {Car myCar = new Car(“Toyota”, “Corolla”, 2020);System.out.println(myCar.displayInfo());}}“`
Through these examples, it is clear that whether in Python, Java, or any other OOP-based language, the essence of classes and objects remains consistent. A class specifies the template, and objects materialize the defined attributes and operations. This modular approach facilitates ease of maintenance, scalability, and reusability in software development, resembling how blueprints aid in constructing numerous similar buildings.
Encapsulation and Information Hiding
Encapsulation is one of the foundational principles of object-oriented programming (OOP). It refers to bundling the data (variables) and the methods (functions) that operate on the data into a single unit, known as a class. This principle ensures that the internal representation of an object is hidden from the outside world. By controlling access to the data inside an object, encapsulation promotes robust security and data integrity.
In practice, encapsulation is achieved through access specifiers such as public, private, and protected. Private access specifiers limit the visibility of a class’s data members and methods to within the class itself. By setting attributes as private, direct modification from outside the class is restricted. This controlled access is accomplished via public methods, also referred to as getter and setter methods. These methods provide a controlled way to retrieve or modify the value of private attributes.
For example, consider a class `BankAccount` with a private attribute `balance`. The balance cannot be directly modified from outside the class. Instead, the class provides public methods such as `deposit()` and `withdraw()` to interact with the balance. This way, the class can enforce rules, such as preventing negative balances or overdrafts, ensuring data integrity.
The advantages of encapsulation in OOP are manifold. Firstly, it promotes modularity, making the code easier to maintain and understand. Changes in the internal implementation of a class do not affect other parts of the system, provided the public interface remains consistent. Secondly, it enhances security by safeguarding the data from unintended interference and misuse. Malicious or erroneous code attempting to alter object state directly is prevented, thus reducing the risk of bugs and vulnerabilities.
In conclusion, encapsulation and information hiding are pivotal to the development of robust, secure, and maintainable software applications. By enforcing controlled access to object data, encapsulation ensures that objects maintain a consistent and correct state, which is crucial for the integrity and reliability of any software system.
Abstraction: Simplifying Complex Systems
In object-oriented programming (OOP), abstraction serves as a crucial concept that simplifies the complexity of systems by focusing on essential qualities and filtering out the non-essential details. Abstraction allows developers to manage complex systems more effectively by reducing the cognitive load required to understand and utilize a particular object or system.
Abstraction operates on the principle of modeling real-world entities into classes. When developers create a class, they identify the most relevant attributes and behaviors of the real-world entity and encapsulate them into a simplified model. This model represents the essential features, providing a clear and coherent structure while omitting irrelevant details. For instance, in creating a class for a “Car,” the relevant attributes might include “make,” “model,” and “engine capacity,” while unnecessary details like the specific material of the engine components can be excluded.
By focusing on critical features, abstraction makes it easier for programmers to handle large and intricate codebases. It allows them to interact with objects at a high level without needing to grasp the entire complexity of the underlying implementation. This leads to more maintainable and scalable code, as changes in the system’s complexity can be managed without altering the fundamental abstraction layers.
Furthermore, abstraction in OOP facilitates greater code reusability. By defining abstract classes and interfaces, developers can create systems that specify common characteristics and behaviors without binding to specific implementations. This enables the implementation of polymorphic behavior, where objects of different types can be treated uniformly based on shared abstract features, significantly enhancing the flexibility of the code.
In essence, abstraction helps in managing and taming complexity by enabling developers to concentrate on what an object should do rather than how it does it. As a result, it provides a robust framework for handling sophisticated software systems in a clear, manageable, and efficient manner.
Inheritance: Reusing Code Efficiently
Inheritance is one of the core principles of Object-Oriented Programming (OOP), allowing new classes, known as derived or child classes, to be created based on existing classes, referred to as base or parent classes. This mechanism enables the child classes to inherit attributes and methods from the parent classes, thereby promoting code reuse and enhancing efficiency in software development.
By leveraging inheritance, developers can create a hierarchical relationship between classes where the child class extends the functionality of the parent class. This hierarchical classification helps in organizing and structuring code in a more manageable way. For example, if a base class named “Animal” has methods like “move” and “eat”, a child class named “Bird” can inherit these methods while adding additional functionalities, such as “fly”. This prevents the redundancy of code and fosters a streamlined programming effort, as common attributes and behaviors are maintained in a single location — the parent class.
The main advantage of inheritance lies in its ability to promote code reuse. Rather than rewriting repetitive code across multiple classes, common functionalities can be abstracted out into a base class and reused by any number of derived classes. This efficiency reduces code duplication, minimizes the risk of errors, and simplifies maintenance. Additionally, using inheritance makes it easier to update or extend applications; changes made to the base class automatically propagate to all derived classes, ensuring consistency across the software system.
However, the misuse of inheritance can lead to significant drawbacks, such as tight coupling between parent and child classes. Tight coupling means that changes in the parent class can have unexpected consequences on all its derived classes, making the system fragile and harder to maintain. Furthermore, improper use of inheritance might result in an inheritance hierarchy that is too deep, leading to confusion and complicating debugging efforts. Therefore, developers must carefully consider the design and necessity of inheritance to avoid potential pitfalls and ensure that it serves the intended purpose of promoting code reuse and efficiency.
Polymorphism: Flexibility in Programming
Polymorphism, derived from the Greek words meaning “many shapes,” is a fundamental concept within Object-Oriented Programming (OOP). It enables objects of different classes to be treated as instances of the same class through a common interface. This principle not only fosters flexibility in programming but also enhances the extensibility of software design.
At the core of polymorphism are two techniques: method overloading and method overriding. Method overloading allows a class to have multiple methods with the same name but different parameter lists. This is particularly useful when an operation needs to be performed in slightly different ways depending on the parameters provided. For instance, a class might have a method “add” that can sum two integers, concatenate two strings, or merge two lists, depending on the parameter types.
Method overriding, on the other hand, involves redefining a method in a subclass that already exists in the parent class. This enables a more specialized implementation of the method in the subclass while maintaining the same name and parameter list as the parent class method. Overriding is a cornerstone of runtime polymorphism or dynamic method dispatch. When an overridden method is called on a reference of the parent class holding an object of the subclass, the subclass’s method is executed. This allows for behavior that is specific to the subclass, creating a more versatile and flexible code structure.
Consider an example where we have a parent class “Shape” with a method “draw()”. Both “Circle” and “Square” are subclasses of “Shape” and each overrides the “draw()” method. When a Shape reference pointing to a Circle or Square object calls the “draw()” method, the respective implementation of the method in Circle or Square is executed. This design not only simplifies function calls but also makes it easy to introduce new shapes with their unique drawing implementations without altering the existing code structure.
Polymorphism enhances the scalability of applications by allowing developers to program to an interface rather than an implementation. This way, objects can be manipulated and extended efficiently, leading to more robust and adaptable software solutions.
Best Practices and Common Mistakes in OOP
Object-oriented programming (OOP) can significantly enhance code readability and maintenance when employed correctly. Following a set of best practices is crucial for effectively utilizing this paradigm. One fundamental guideline is to design classes with a single responsibility. Each class should encapsulate a single functionality to adhere to the Single Responsibility Principle (SRP), making the code easier to understand and modify.
Moreover, managing dependencies is essential. Dependency Injection (DI) can aid in reducing tightly coupled components, fostering flexibly and testable code. Rather than creating dependencies directly within classes, inject them, which promotes a more modular and scalable architecture.
Inheritance is a powerful feature in OOP, but its overuse can lead to complex and unmanageable hierarchies. Prefer composition over inheritance to enhance component reuse without inflating class inheritance trees. With composition, objects are constructed using other objects, allowing for more flexible code modification.
Another critical aspect of OOP best practices involves naming conventions. Use descriptive and meaningful names for classes, methods, and variables. Poor naming conventions can obscure the purpose and functionality of code, leading to increased difficulty in debugging and maintaining it. Consistent and clear naming improves comprehension and collaboration among development teams.
Additionally, avoid the common pitfall of creating overly large classes, known as “God Objects.” These classes tend to handle too many responsibilities, undermining modularity and increasing the complexity of the system. Break down functionalities into smaller, manageable classes to adhere to the Single Responsibility Principle and promote better code organization.
Finally, it is vital to decide when to use OOP versus other programming paradigms, such as procedural or functional programming. OOP excels in scenarios requiring complex abstractions and manageable, reusable components, while procedural may be more efficient for straightforward, linear tasks. Functional programming can simplify code with stateless operations and higher-order functions.