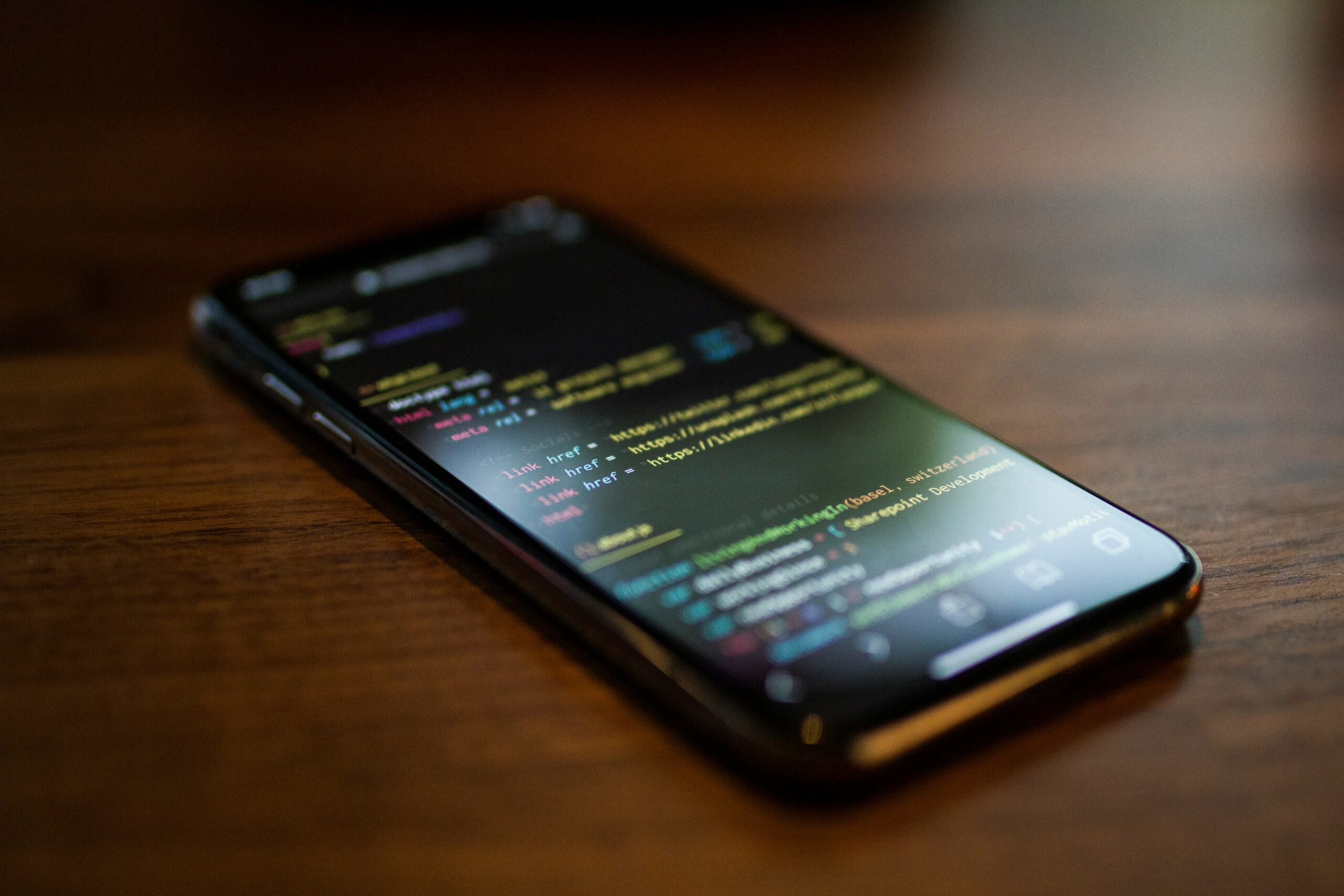
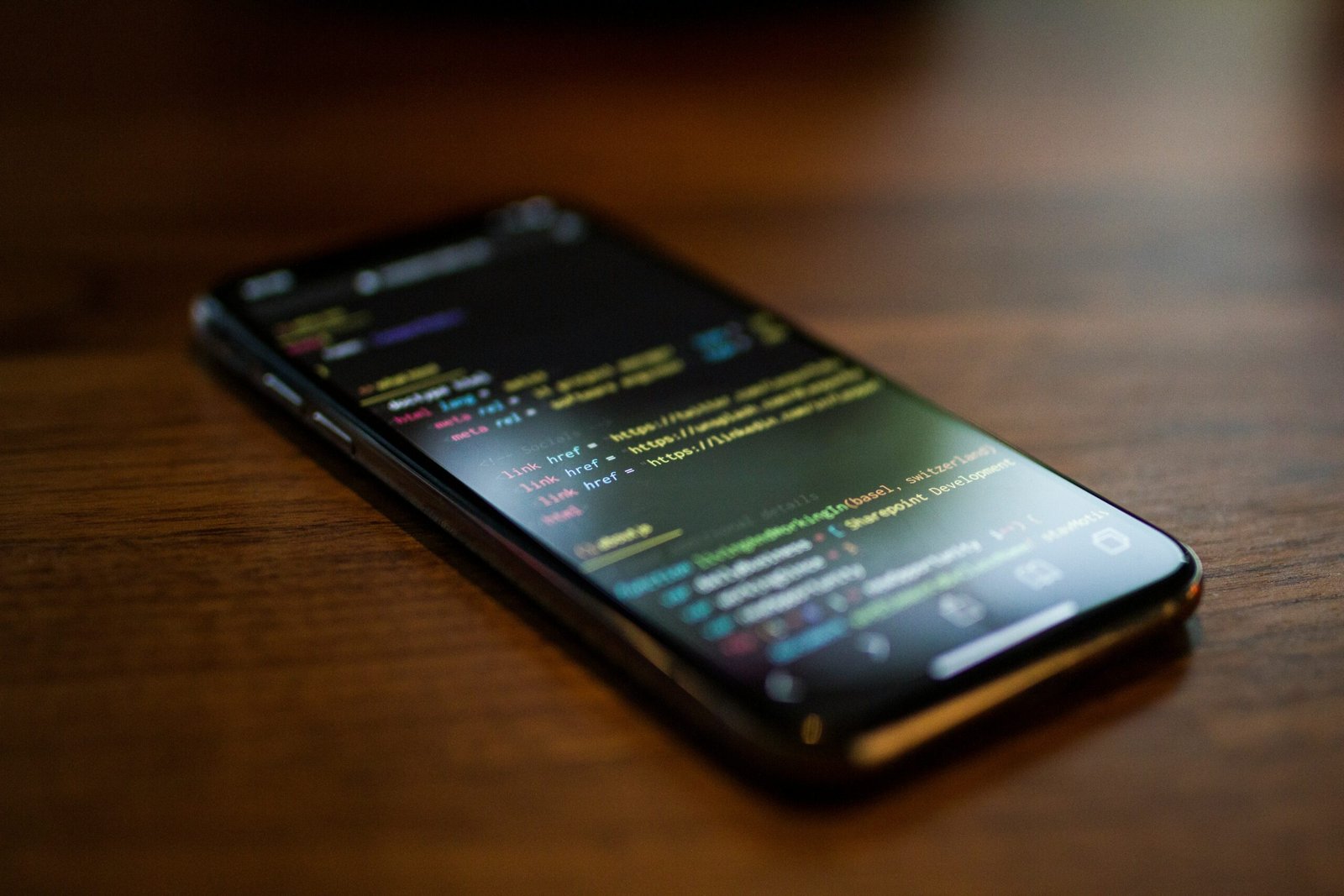
Introduction to Java
Java, a high-level, class-based programming language, has been a cornerstone of software development since its inception. Conceived by James Gosling and developed at Sun Microsystems, Java made its debut in 1995. Originally designed to have as few implementation dependencies as possible, Java aimed to embody the principle of “write once, run anywhere,” allowing compiled Java code to run on any platform that supports Java without the need for recompilation.
Java’s creation stemmed from the need for a versatile and robust programming language that could cater to the evolving landscape of networked environments. Since its first release, Java has undergone significant transformation and evolution. The language has transitioned through numerous versions, each adding enhancements and refining features while maintaining backward compatibility. Major milestones include the release of Java 2 in 1998, which introduced the concept of the Java Virtual Machine (JVM), and Java 5 in 2004, which brought in generics, enumerated types, and metadata.
The impact of Java on the software development world has been profound. Its inherent simplicity and portability have contributed to its widespread adoption across various applications, from mobile devices to large-scale enterprise systems. Java’s robust performance, portability, and extensive libraries have equipped developers to create highly reliable and efficient applications. Over the years, Java has also expanded its ecosystem with integrated development environments (IDEs) like Eclipse and IntelliJ IDEA, which have further streamlined and enhanced its programming experience.
Currently, Java continues to be a dominant force in the industry. With regular updates and the introduction of new features, Java remains an essential skill for developers and a critical component in many business infrastructures. Its longevity and ongoing development efforts ensure that Java stands as a vital tool in the ever-evolving field of software development.
Key Features of Java
Java stands out in the programming world due to its robust and versatile features, making it a favored choice amongst developers. One of the most notable traits of Java is its object-oriented nature, which promotes modularity and code reuse. By utilizing objects and classes, Java allows developers to create well-structured, maintainable code.
A significant feature that enhances Java’s appeal is its platform independence. The Java Virtual Machine (JVM) plays a crucial role in this aspect, enabling Java applications to run on any device with a JVM installed, regardless of the underlying hardware and operating system. This “write once, run anywhere” capability streamlines the development process and broadens potential deployment environments.
Automatic memory management is another cornerstone of Java’s design. Through its Garbage Collection mechanism, Java effectively handles memory allocation and deallocation, reducing the risk of memory leaks and improving application performance. This allows developers to focus more on core programming tasks rather than managing memory manually.
Java excels in multi-threading capabilities, which facilitates the concurrent execution of two or more threads. This feature allows more efficient utilization of CPU resources, enhancing the performance of complex and resource-intensive applications. Developers can thus design applications that handle multiple tasks simultaneously, improving both responsiveness and throughput.
Security has always been a priority for Java. The language incorporates several advanced security features such as bytecode verification, secure class loading, and a comprehensive security manager, which collectively provide a robust framework for developing secure applications. Java’s security model helps to protect systems from malicious code and data breaches effectively.
Moreover, Java is revered for its simplicity in syntax. Its straightforward syntax, which closely resembles natural language, allows for easier learning and faster coding. This simplicity not only aids beginners in grasping fundamental programming concepts quickly but also helps experienced developers to produce clean, efficient, and readable code.
Overall, these primary features make Java a compelling choice for developing a wide range of applications, from desktop software to web and mobile applications, ensuring its continued popularity in the software development industry.
The Java Ecosystem
The Java ecosystem is an expansive suite that encompasses various tools, libraries, and platforms designed to bolster the development, execution, and management of Java applications. Central to this ecosystem are three pivotal components: the Java Development Kit (JDK), the Java Runtime Environment (JRE), and the Java Virtual Machine (JVM). Understanding how these components interplay is essential for maximizing the efficiency and portability of Java programs.
The Java Development Kit (JDK) is a comprehensive toolkit that provides developers with the necessary resources to develop Java applications. It includes the Java compiler, the Java application launcher, and a rich set of libraries and tools, such as the debugger and documentation generator. By facilitating the compilation of Java source code into bytecode, the JDK plays an indispensable role in the initial stages of Java application development.
The Java Runtime Environment (JRE) complements the JDK by offering a runtime environment in which Java applications can execute. The JRE encompasses the essential libraries, Java Virtual Machine, and other components required to run Java programs. It does not include the development tools found in the JDK, making it a more streamlined package for end-users who need to run Java applications without the overhead of development resources.
At the heart of the Java ecosystem is the Java Virtual Machine (JVM). The JVM serves as an abstract computing machine that enables Java applications to be executed on any device or operating system, given that there is a compatible JVM available. It translates Java bytecode into machine-specific instructions, thereby underpinning Java’s “write once, run anywhere” paradigm. Furthermore, the JVM provides crucial performance optimizations, such as Just-In-Time (JIT) compilation and garbage collection, which enhance the execution speed and memory management of Java applications.
The seamless integration of the JDK, JRE, and JVM is instrumental in fostering the development and execution of robust Java applications. Each component plays a unique role, yet their synergistic relationship ensures that Java applications are efficient, portable, and scalable. This triad forms the backbone of the Java ecosystem, empowering developers to create versatile solutions across diverse computing environments.
Getting Started with Java
For beginners aiming to delve into Java programming, setting up the right tools and environments is crucial. The first step involves installing the Java Development Kit (JDK), which is necessary for compiling and running Java code. Oracle’s official website offers the latest JDK version, which can be downloaded and installed following their straightforward instructions.
Once the JDK is in place, choosing the right Integrated Development Environment (IDE) can enhance productivity. Popular choices include IntelliJ IDEA, Eclipse, and NetBeans, each offering distinct features tailored to different programming needs. To begin with IntelliJ IDEA, download the Community Edition, install it, and configure the JDK path. Eclipse and NetBeans follow a similar setup process, involving downloading their respective packages and configuring the environment settings.
With the development environment ready, the next step is writing a simple program. The canonical “Hello, World!” application serves as a perfect starting point. Here’s what a basic Java code looks like:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Breaking down this program, one can observe the structure of a Java application. The public class
keyword declares a class named HelloWorld. Inside this class, the main
method serves as the entry point of any Java application. The code System.out.println("Hello, World!");
outputs the string “Hello, World!” to the console, demonstrating basic syntax and the use of pre-defined classes in Java.
Understanding the foundational structure and syntax is key for progressing in Java programming. Initially, it’s essential to grasp basic concepts such as the structure of classes, methods, and statements. Coupled with practice and exploration of more advanced topics, these fundamentals will pave the way for mastering Java.
Object-Oriented Programming in Java
Object-Oriented Programming (OOP) is a paradigm central to Java, providing a robust framework for building modular and reusable code. OOP is based on several key principles: classes, objects, inheritance, polymorphism, abstraction, and encapsulation. Together, these concepts form the backbone of Java’s approach to software development.
Classes and Objects: In Java, a class serves as a blueprint for objects. A class defines properties (attributes) and behaviors (methods) that its objects will have. For example, a class Car
might have attributes like color
and model
, and methods like drive()
and brake()
. An object is an instance of a class. Creating an object of the Car
class would allow you to interact with the specific car’s properties and methods.
Inheritance: This concept allows a new class (subclass) to inherit properties and methods from an existing class (superclass). Inheritance promotes code reusability by enabling common functionality to be shared across multiple classes. For instance, if there’s a superclass Vehicle
, the Car
and Bike
classes might inherit from it, inheriting attributes like speed
and methods like stop()
.
Polymorphism: Polymorphism allows objects to be treated as instances of their parent class rather than their actual class. It is achieved through method overriding and method overloading. Polymorphism is advantageous because it enables a single interface to represent different underlying forms (data types). For example, a Vehicle
type object might refer to an instance of a Car
or a Bike
, and a move()
method might behave differently for each subclass.
Abstraction: Abstraction focuses on hiding the complex implementation details and showing only the essential features of an object. Java uses abstract classes and interfaces to achieve abstraction. An abstract class cannot be instantiated and may contain abstract methods that subclasses must implement. Interfaces define a contract of methods that implementing classes must fulfill, thereby fostering a standard structure across different parts of an application.
Encapsulation: Encapsulation involves wrapping the data (attributes) and code (methods) into a single unit, typically a class, and restricting access to some components. This is done by declaring class fields as private and providing public getter and setter methods to access and update these fields. Encapsulation helps protect the integrity of an object’s state by preventing unauthorized access or modification.
Incorporating these OOP principles allows developers to build well-organized, maintainable, and scalable Java applications. By adhering to the principles of OOP, Java programs become easier to debug, extend, and refactor, fortifying them against the challenges of complex software development.
Java Standard Libraries and APIs
The Java programming language boasts a robust collection of standard libraries and APIs, which are fundamental for a wide array of functionalities – from data manipulation to network communication and more. These resources provide predefined classes and interfaces that make development more efficient and streamlined. Among the numerous libraries and APIs available in Java, some of the most commonly used include java.util
, java.io
, java.net
, and java.sql
.
java.util: The java.util
package encompasses a broad range of utilities and collections which are indispensable for developers. This package includes classes like ArrayList
, HashMap
, Date
, and Calendar
. These classes support data structures and algorithms, providing essential methods for searching, sorting, and manipulating data efficiently. For example, ArrayList
allows dynamic array manipulation, while HashMap
enables key-value pairings, widely used for data retrieval operations.
java.io: Handling input and output operations is made seamless with the java.io
package. This library includes classes for reading and writing data to files, streams, and network connections. Notable classes such as FileReader
, FileWriter
, BufferedReader
, and PrintWriter
, facilitate efficient reading and writing of data. For instance, BufferedReader
can be utilized for reading text from a character-input stream, efficiently buffering characters to provide efficient reading of text data.
java.net: Networking capabilities are well-supported through the java.net
package, which includes classes for network communication. This package facilitates the creation of client-server models and the establishment of socket-based communication. Classes such as Socket
, ServerSocket
, and URL
are crucial for building network applications. For example, the Socket
class can be used to connect to a specific server, and the ServerSocket
class allows for the creation of server-side programs that can accept client requests.
java.sql: Database operations are streamlined with the java.sql
package, a key component of Java’s ability to interact with relational databases. This package includes classes and interfaces like Connection
, Statement
, ResultSet
, and PreparedStatement
. These classes are pivotal in executing SQL commands, managing database connections, and retrieving query results. For example, the Connection
interface manages the connection between Java applications and databases, while PreparedStatement
is used for executing parameterized queries, enhancing security by preventing SQL injection.
In a nutshell, these standard libraries and APIs vastly extend the capabilities of the Java programming language, enabling developers to build robust, efficient, and high-performing applications. By providing essential functionalities out-of-the-box, they allow developers to focus on crafting innovative solutions without getting bogged down in reinventing fundamental operations.
Java Applications and Use Cases
Java, a versatile and robust programming language, has cemented its place in various domains of software development. Its ability to create efficient and scalable applications makes it an irreplaceable asset in the modern tech ecosystem. One of the primary areas where Java shines is in enterprise applications. Companies across the globe leverage Java to build large-scale, mission-critical systems. Java’s object-oriented nature and extensive libraries enable it to handle complex business logic and integration with other systems effortlessly.
In the realm of web development, Java has a strong foothold through JavaServer Pages (JSP), Servlets, and frameworks like Spring and Hibernate. These technologies enable developers to create dynamic and secure web applications. The Spring framework, in particular, is lauded for its dependency injection and aspect-oriented programming features, which streamline development and contribute to maintainability.
The mobile application landscape, especially concerning Android development, sees Java as a cornerstone. Android, the world’s most popular mobile operating system, uses a modified version of Java to build its applications. Java’s platform independence and performance optimization are critical for developing Android apps that perform well on a plethora of devices with varying specifications.
Another significant domain where Java exhibits its prowess is in scientific computing. Java’s robust mathematical libraries, such as Apache Commons Math and JScience, enable scientists and engineers to perform complex calculations and simulations efficiently. Its cross-platform capabilities ensure that the same Java code can run seamlessly on different operating systems, which is vital in research environments.
In the era of big data, Java continues to be a preferred choice for developing data processing and analytics tools. Technologies like Apache Hadoop and Apache Spark, which are instrumental in handling large data sets, are built using Java. These tools enable organizations to process and analyze massive amounts of data quickly, which is pivotal in making informed business decisions.
Prominent companies and products that rely heavily on Java include LinkedIn, which uses Java for its backend services, eBay, which utilizes Java for its extensive online auction platform, and NASA, which employs Java for various space exploration missions. These case studies exemplify how Java’s reliability and scalability make it indispensable for high-profile projects.
Future of Java
The future of Java looks promising as the language continues to evolve, driven by a robust community and Oracle’s consistent updates. The ongoing development ensures that Java remains versatile and relevant in a rapidly changing technological landscape. As we look ahead, several factors stand out that could shape Java’s trajectory in the software development industry.
One of the most significant components is the introduction of new features in upcoming Java versions. Project Loom, for example, aims to simplify concurrency models and enhance performance by reducing the complexity of writing scalable applications. Such advancements promise to make Java even more efficient and developer-friendly.
Another critical area is Project Panama, which focuses on improving the interface between Java and native code. Enhancements in this domain could broaden Java’s applicability, particularly in performance-intensive applications like scientific computing and high-frequency trading. These updates exemplify how ongoing innovations are set to expand Java’s utility beyond its traditional use cases.
The open-source community plays a vital role in driving Java’s evolution. Contributions from developers around the world ensure that Java continuously adapts to new challenges and opportunities. Collaboration through platforms like OpenJDK fosters a diverse ecosystem where innovative ideas can thrive, further reinforcing Java’s position as a top-tier programming language.
Emerging trends and technologies are poised to influence how Java is utilized in the future. The growth of cloud computing and microservices architecture, for instance, necessitates languages that offer scalability and robust security features. Java’s adaptability makes it well-suited for these environments. Additionally, with the rise of machine learning and artificial intelligence, Java’s stability and vast library support could prove beneficial for developers exploring these fields.
In summary, Java’s future is bright, underpinned by continuous improvements and a vibrant community. As it adapts to emerging trends and technologies, Java is expected to remain a significant player in the software development industry, offering both reliability and innovation.