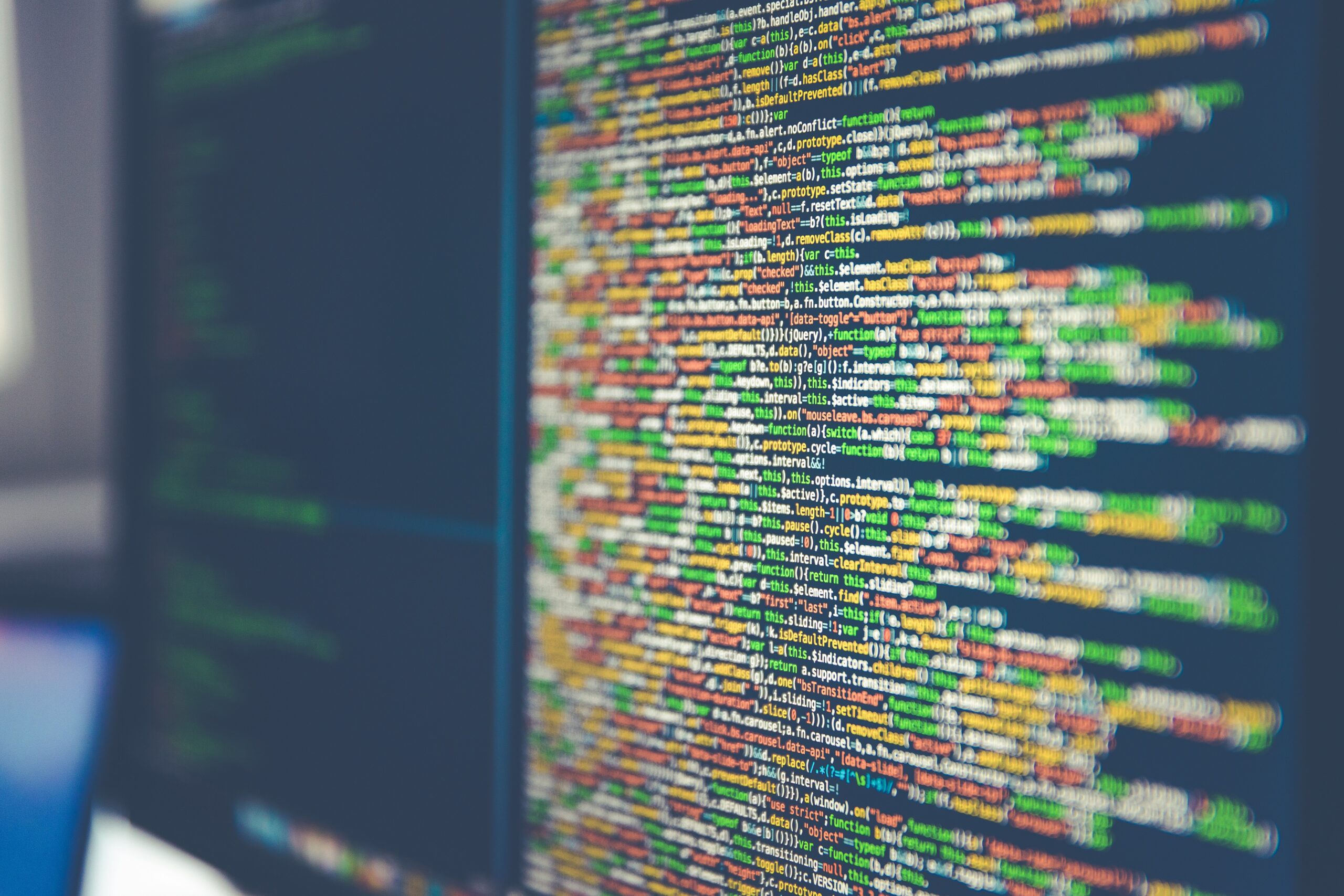
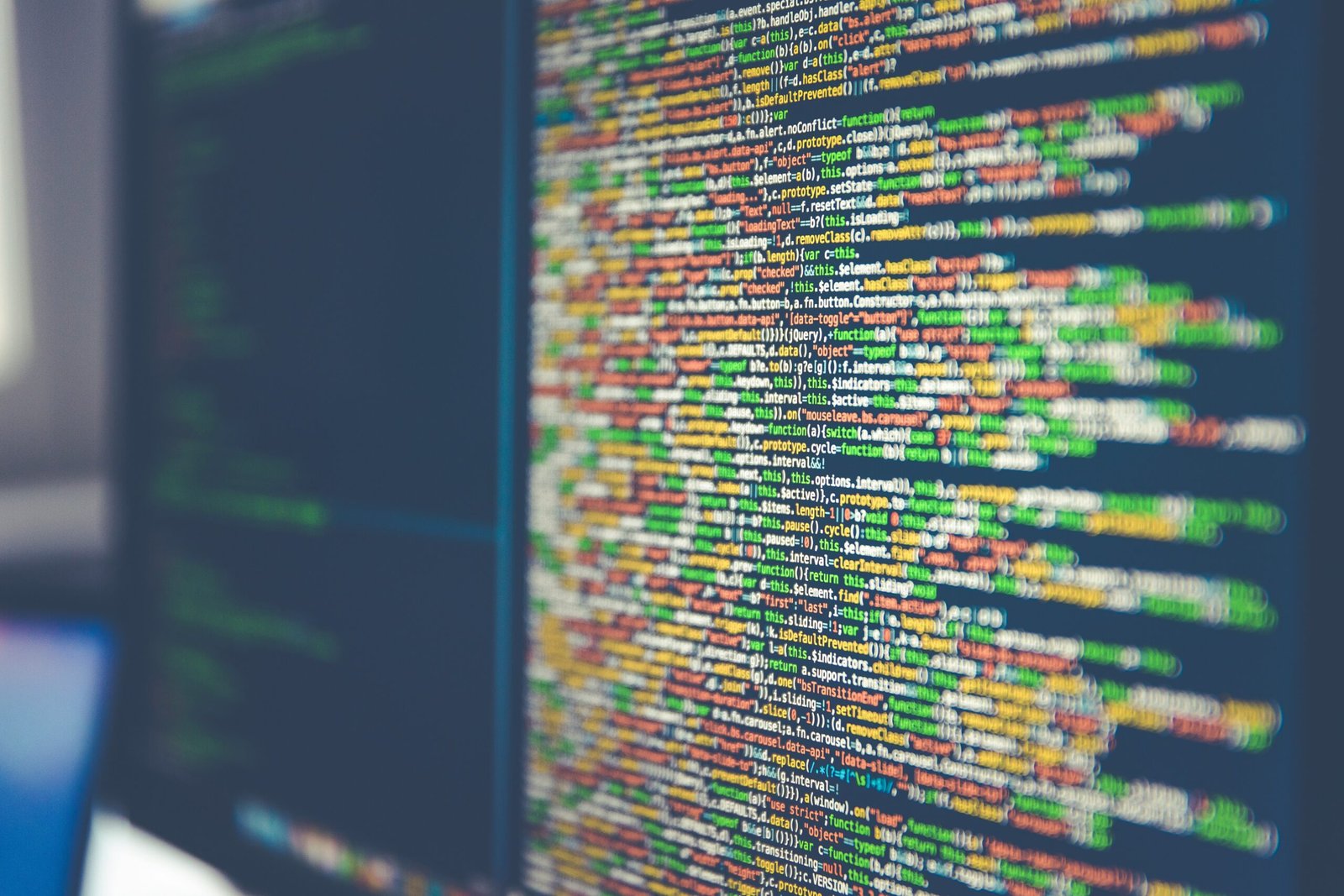
Introduction to Imperative Programming
Imperative programming stands as one of the fundamental paradigms in computer science, representing a model for structuring and writing software that closely mirrors how a computer’s hardware executes tasks. Originating in the mid-20th century, imperative programming has profoundly shaped the development and evolution of programming languages, and remains integral to understanding modern-day software engineering. At its core, imperative programming entails the use of statements to change a program’s state through commands and instructions. The programmer provides explicit directions, step-by-step, specifying “how” a task should be accomplished, rather than “what” the outcome should be.
The historical roots of imperative programming can be traced back to some of the earliest programming languages, such as Fortran and Cobol. These languages were built to meet the needs of numerical computation and business data processing, respectively. Over time, the paradigm has evolved to include more sophisticated constructs and abstractions seen in languages such as C, Java, and Python. Imperative programming languages typically feature constructs like loops, conditionals, and variable assignments, which are pivotal for procedural task execution.
Imperative programming’s significance lies in its directness and efficiency. By providing a clear and detailed sequence of operations, it aligns closely with the underlying machine code executed by the processor, thereby enabling optimization and fine-control over resource management. This characteristic is crucial for developing performance-critical applications, from operating systems to high-performance computing solutions.
Throughout this blog post, we will delve deeper into the essential characteristics of imperative programming, dissecting its syntax and exploring its advantages and limitations. Additionally, comparative discussions with other programming paradigms will aid in highlighting its unique features and utility. By understanding these foundational elements, aspiring programmers and seasoned developers alike can leverage imperative programming to create robust, efficient, and maintainable software systems.
Characteristics of Imperative Programming
Imperative programming is a paradigm that revolves around explicit commands for the computer to perform. One of the most defining characteristics of imperative programming is the direct manipulation of computer memory. Programmers explicitly instruct the computer on how to manipulate memory by specifying the exact steps required. This gives them fine-grained control over how memory is allocated and managed, often leading to more efficient use of resources.
Another hallmark of imperative programming is the explicit sequencing of commands. In an imperative program, instructions are given in a specific order, and the program’s flow of control strictly follows this order. This sequence of commands results in state changes as the program runs, with each instruction potentially altering the system’s state. This contrasts with other paradigms, where the order of operations may be abstracted away, and state changes are managed differently.
State changes are a core aspect of imperative programming. The state of a program can be thought of as the stored information at any given point in its execution. In an imperative approach, state changes are explicitly coded by the programmer through variable assignments, conditionals, and loops. The programmer must manage and keep track of the state throughout the program’s lifecycle, which can be both powerful and demanding.
When comparing imperative programming to other paradigms, such as declarative and functional programming, its uniqueness becomes evident. Declarative programming focuses on the ‘what’ rather than the ‘how’. It abstracts the control flow and state management, allowing the programmer to specify the desired outcomes without detailing the steps to achieve them. Functional programming, a subset of declarative programming, employs immutable data and pure functions, minimizing state changes and side effects.
In summary, the primary characteristics of imperative programming include direct memory manipulation, explicit command sequencing, and state changes. While this paradigm offers precise control, it requires meticulous management of system resources and program state, setting it apart from declarative and functional approaches.
Key Concepts in Imperative Programming
Imperative programming is a paradigm centered around the concept of changing a program’s state through explicit commands. One of the fundamental building blocks in this paradigm is the variable. Variables serve as storage locations that a program can use to retain data that might change during execution. They allow for dynamic allocation and manipulation of data, making them essential to the operation of imperative code.
Control structures are another critical element in imperative programming. These include loops and conditional statements, which manage the flow of execution. Loops, such as ‘for’, ‘while’, and ‘do-while’, enable repetitive execution of code blocks until a particular condition is met. This repetitive execution is crucial for tasks requiring iteration over data sets. Conditionals, like ‘if’, ‘else if’, and ‘else’, facilitate decision-making in code. By evaluating Boolean expressions, conditionals can lead the program to execute different code paths based on the outcomes of these expressions.
Procedures and functions further enrich the capabilities of imperative programming languages. These are modular blocks of code designed to perform specific tasks, which can be reused throughout a program. Procedures (often referred to simply as functions in many modern languages) encapsulate code into callable units, promoting code reusability and modularity. By passing arguments to these functions, developers can create flexible and maintainable code structures.
The interplay of these elements—variables, control structures, and procedures/functions—constitutes the foundation of imperative programming. Developers use these tools to write code that tells the computer ‘how’ to perform tasks by changing the program’s state in a specified sequence. This approach contrasts with declarative paradigms, where the focus is on ‘what’ the program should accomplish rather than ‘how’ it should be done. Understanding these key concepts is essential for mastering imperative programming and effectively manipulating program state over time.
Popular Imperative Programming Languages
Imperative programming languages have been the backbone of software development for decades. Among the most prominent languages in this paradigm are C, C++, Python, and Java. Each of these languages brings unique characteristics and historical significance, making them highly favored for various applications.
C, developed in the early 1970s by Dennis Ritchie at Bell Labs, is often considered one of the foundational languages in imperative programming. Its ability to provide low-level memory access and fine control over system resources has made it indispensable in system programming, operating systems, and embedded systems. Features like pointers, manual memory management, and a simple syntax contribute to its efficiency and performance, ensuring its ongoing widespread use.
Building on the strengths of C, C++ was introduced by Bjarne Stroustrup in the 1980s. C++ extends C by adding object-oriented features without compromising the imperative style of programming. This blend has facilitated the development of complex software systems, including games, GUI-based applications, and real-time simulations. The ability to use classes and inherit properties while maintaining procedural control gives developers the flexibility to create robust and maintainable code.
Python, created by Guido van Rossum in the late 1980s, is a versatile language that has gained immense popularity. While often associated with scripting and rapid application development, Python remains fundamentally imperative. Its simple, readable syntax and powerful string handling make it an excellent choice for beginners and experienced developers alike. From web development and automation scripting to data analysis and artificial intelligence, Python’s extensive standard library and ecosystem offer tools for various imperative programming tasks.
Java, introduced by Sun Microsystems in the mid-1990s, brought the concept of write-once, run-anywhere to the forefront of software development. Java’s strong typing, automatic garbage collection, and extensive standard library make it particularly suited for large-scale enterprise applications. Its platform independence, achieved through the Java Virtual Machine (JVM), has enabled developers to build robust, scalable applications that run consistently across diverse environments.
In summary, C, C++, Python, and Java epitomize the principles of imperative programming while catering to diverse development needs. Their distinct features and capabilities continue to empower developers to write efficient, reliable, and optimized code, cementing their positions as essential tools in the world of software engineering.
Advantages of Imperative Programming
Imperative programming offers several noteworthy advantages, making it a favorable choice for many applications. One of the primary benefits is its ease of understanding, especially for beginners. Since imperative programming focuses on specifying explicit instructions that a computer must follow, it aligns closely with the conventional sequential thought process. This characteristic simplifies the learning curve for newcomers, enabling them to grasp programming fundamentals more efficiently.
Another significant advantage is the potential for improved performance. Imperative programming allows developers to manipulate system resources directly, providing fine-grained control over the computer’s operations. This control can lead to optimized performance because developers can specify how memory, CPU resources, and input/output operations are managed. In applications where performance is critical, such as real-time systems, game development, or scientific computations, imperative programming can offer distinct advantages.
Moreover, imperative programming excels in its ability to create detailed and specific solutions. The explicit nature of imperative languages means developers can craft highly tailored instructions to tackle complex problems. For example, when building a sorting algorithm, an imperative approach allows coders to specify each step – from comparing elements to exchanging positions – ensuring precision and clarity in the solution.
Real-world examples illustrate these advantages effectively. Consider the case of system programming, where performance and resource management are paramount. Languages like C, a quintessential imperative language, are often used to develop operating systems and embedded systems due to their ability to offer direct hardware manipulation and optimized performance.
Thus, its ease for beginners, enhanced performance capabilities, and precision in problem-solving make imperative programming a powerful paradigm in software development.
Disadvantages and Challenges
Imperative programming, while widely used and deeply ingrained in software development, is not without its challenges and disadvantages. One of the primary difficulties is managing state and side effects. In imperative programming, the state of a program is continuously changing through variable assignments and state mutability. This can lead to unintended consequences as any change in the program’s state can produce side effects that are difficult to anticipate and debug.
Another significant challenge is the complexity associated with large codebases. As the size of the imperative program grows, so does the intricacy of its control flow and state management. This complexity makes it hard to track which part of the state is affected by which piece of code, leading to potential conflicts and bugs that are arduous to resolve. Developers often find themselves dealing with “spaghetti code,” where the flow of control weaves in and out in a tangled mess, complicating maintenance and further development.
Issues with readability and maintainability also pose substantial hurdles in imperative programming. The low-level operations and detailed control over the computer state, while powerful, can make the code less intuitive to read and understand. The emphasis on how a task is accomplished, rather than what the task is, often requires more effort to comprehend the logic behind the code, particularly for those unfamiliar with the codebase or imperative paradigm.
Comparatively, other programming paradigms such as functional programming offer potential solutions to these challenges. Functional programming, for instance, emphasizes immutability and first-class functions, reducing the potential for side effects and enhancing code readability and maintainability. Similarly, object-oriented programming organizes code into modular, reusable objects, which can simplify state management and code comprehension.
Overall, while imperative programming is powerful and versatile, it comes with specific disadvantages and challenges that require careful consideration, particularly in the context of managing state, handling complexity in large codebases, and ensuring readability and maintainability. By understanding these issues and comparing them with alternative paradigms, developers can make more informed decisions about the most suitable approaches for their projects.
Imperative vs. Declarative Programming
Imperative programming and declarative programming represent two fundamentally different approaches to writing code. While imperative programming focuses on outlining the steps needed to achieve a desired outcome, declarative programming emphasizes what needs to be achieved without specifying how to achieve it.
Declarative programming centers around the principle of describing the desired results. In this paradigm, a programmer articulates the logic of computation without explicitly describing its control flow. Common examples of declarative languages include SQL for database queries and HTML for web page structure. In these languages, rather than detailing the specific steps to retrieve data or display elements, a programmer simply defines the criteria or structure.
The key differences between imperative and declarative programming lie in how they manage execution flow and how they describe tasks. Imperative programming uses statements to change a program’s state through sequential steps, loops, and conditionals. Conversely, declarative programming uses expressions and declarations to convey what the program should accomplish, leaving the “how” to the underlying system.
To illustrate these differences, consider the task of calculating the sum of a list of numbers. In an imperative style, the code might look like this:
let sum = 0;for (let i = 0; i < numbers.length; i++) {sum += numbers[i];}
This example clearly illustrates the step-by-step instructions for iterating through the array and updating the sum. Now, consider the same task using a declarative style in a functional programming language:
const sum = numbers.reduce((acc, num) => acc + num, 0);
In this example, the reduce
function abstracts away the iteration details, allowing the programmer to succinctly describe the desired result: the sum of the array. Instead of focusing on the control flow, the declarative approach prioritizes the final outcome.
While declarative programming can simplify and abstract complex logic, imperative programming provides fine-grained control over the sequence of operations. Both paradigms have their strengths and are often used together within a software solution to leverage the benefits of each approach effectively.
Practical Applications of Imperative Programming
Imperative programming is widely used across various fields due to its straightforward approach to managing the state of a program through explicit commands. One of the most prominent domains where imperative programming thrives is system programming. In systems programming, close interaction with hardware resources and efficient memory management are crucial. Imperative languages, such as C and Assembly, offer fine-grained control over system resources, making them ideal for developing operating systems, device drivers, and embedded systems. The Linux operating system, for instance, is primarily written in C, showcasing the performance and control that imperative programming can provide.
Another significant application of imperative programming is in game development. Games often require real-time performance, detailed control over hardware, and efficient memory usage, aspects where imperative languages excel. C++ is a popular choice in the gaming industry, enabling developers to create complex algorithms for rendering, physics simulations, and handling user input. The Unreal Engine, one of the leading game development platforms, leverages C++ to deliver high-fidelity graphics and responsive gameplay, illustrating the practical benefits of imperative programming in action.
Imperative programming is also favored in performance-critical applications where execution speed and resource management are paramount. Examples include scientific computing, financial modeling, and high-frequency trading. In these scenarios, the predictability and efficiency of imperative languages like Fortran and C ensure that complex computations are performed swiftly and accurately. NASA, for example, employs Fortran for many of its computationally intensive simulations, benefiting from the language’s optimization capabilities.
Real-world case studies further highlight the efficacy of imperative programming. One notable example is Google’s use of C++ in the development of its Chromium browser. The choice of C++ enables the browser to deliver superior speed and resource management, crucial for handling multiple tabs and extensions without degrading performance. Similarly, in the context of high-frequency trading, firms often rely on C++ to manage substantial data volumes and execute trades at microsecond intervals effectively.