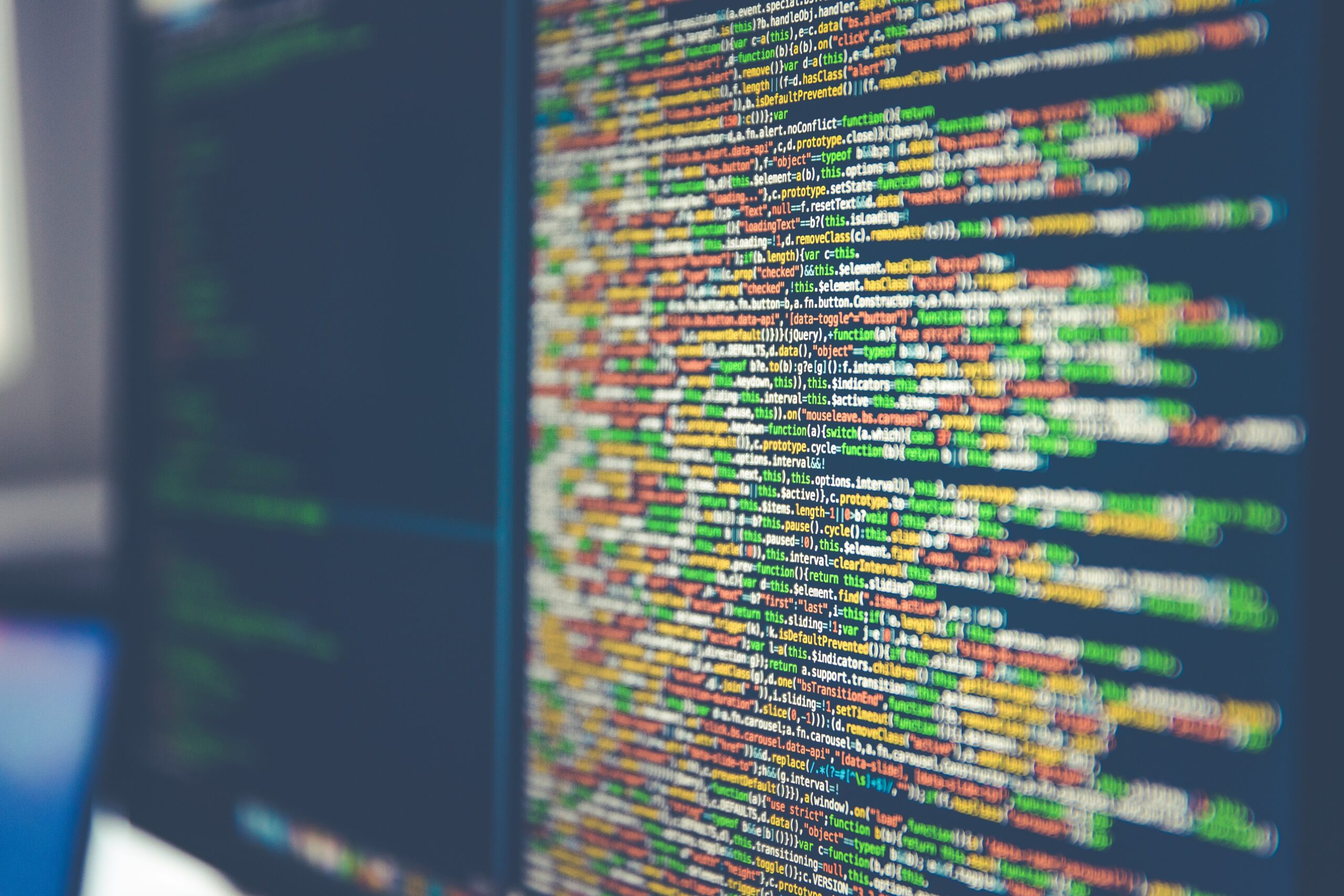
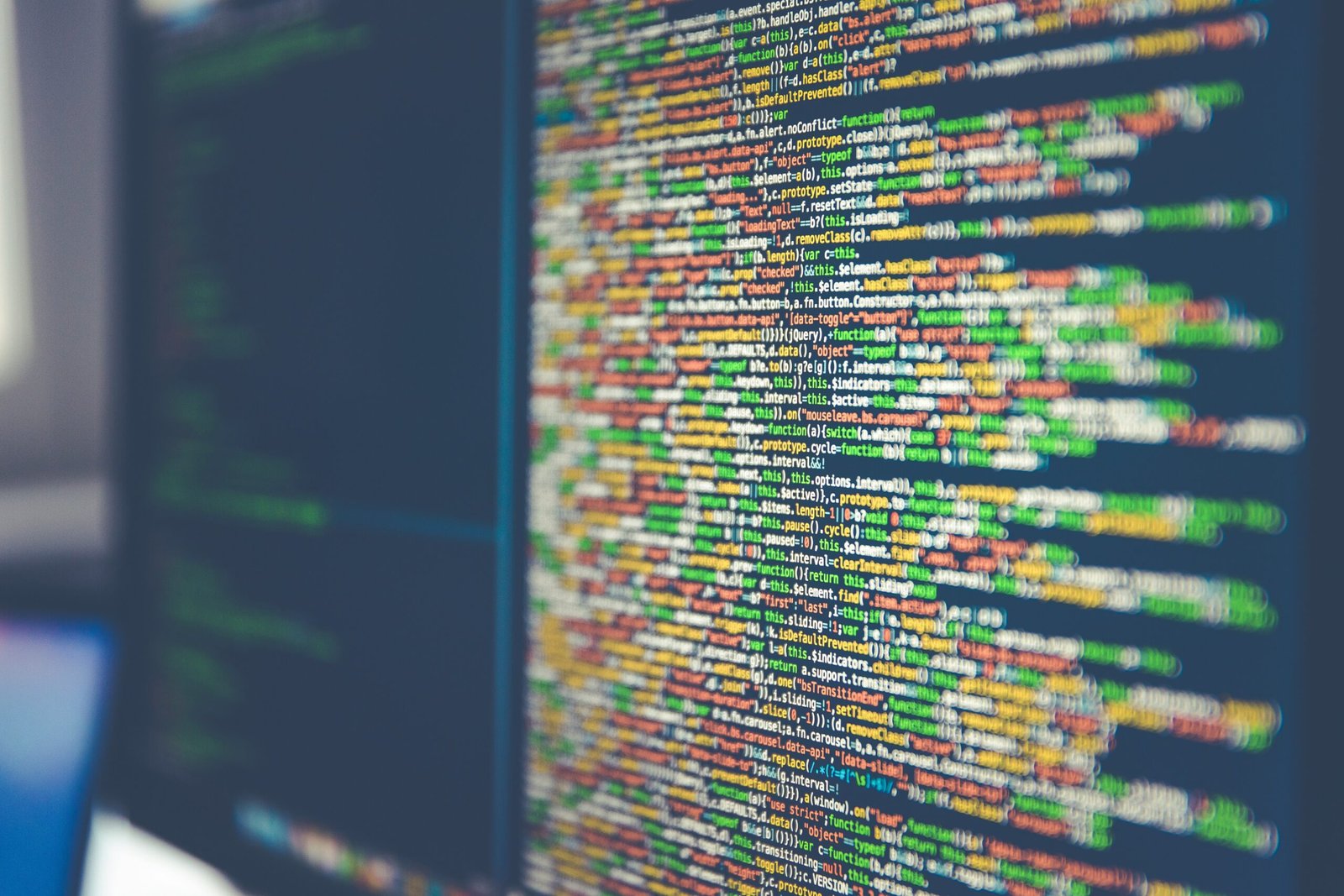
Functional programming is a paradigm that focuses on the use of pure functions to build software. Originating from mathematical principles, functional programming is deeply rooted in the concepts of lambda calculus, a formal system devised in the 1930s by mathematician Alonzo Church. This paradigm treats computation as the evaluation of mathematical functions and avoids changing state or mutable data, which differentiates it from imperative programming.
Lambda calculus forms the foundation of functional programming by providing a universal model of computation based on function abstraction and application. In this model, functions are first-class citizens, meaning they can be passed as arguments, returned by other functions, and assigned to variables. This mathematical rigor allows for more predictable and modular code, making it easier to reason about and test software components.
Functional programming has experienced a resurgence in popularity in recent years, driven by the growing need for concurrent and parallel processing in modern software applications. As multi-core processors and distributed systems become more prevalent, the ability to efficiently manage concurrent tasks has become crucial. Functional programming’s emphasis on immutability and stateless functions naturally lends itself to concurrent execution, reducing the complexity associated with synchronization and shared state.
The adoption of functional programming languages such as Haskell, Scala, and Elixir, as well as the incorporation of functional paradigms in mainstream languages like JavaScript and Python, highlights the paradigm’s relevance and utility. By understanding the principles and practices of functional programming, developers can write more reliable, maintainable, and scalable software, capable of meeting the demands of contemporary computing environments.
Core Concepts of Functional Programming
Functional programming revolves around several core principles that distinguish it from other programming paradigms. Among these, immutability, first-class functions, higher-order functions, and pure functions are particularly significant.
First and foremost is the concept of immutability. In functional programming, data is immutable, meaning it cannot be altered after it is created. This principle ensures that variables remain constant, enhancing predictability and reducing potential side-effects. For example, consider a list of numbers. Rather than modifying the list directly, you’d generate a new list with the desired modifications, leaving the original list unchanged.
Next, first-class functions are fundamental in functional programming. This means that functions are treated as first-class citizens – they can be passed as arguments, returned from other functions, and assigned to variables. This offers greater flexibility and modularity in your code. For instance, a function that doubles a number can be passed to another function that applies it to each element in an array.
Closely related are higher-order functions, which either take other functions as arguments or return them. These functions allow for the development of more abstract and reusable code components. A classic example is the `map` function, which applies a given function to each item in a collection, returning a new collection with the results without mutating the original data structure.
Pure functions are another vital concept. A pure function is one that, given the same inputs, will always produce the same output and has no side effects. This predictability and stability make debugging and testing more straightforward. An example of a pure function is a simple mathematical operation, like adding two numbers together – the output depends solely on the input values and nothing else.
These concepts – immutability, first-class functions, higher-order functions, and pure functions – form the foundation of functional programming. By adhering to these principles, developers can produce more reliable, maintainable, and robust code.
Benefits of Functional Programming
Functional programming has garnered attention for its numerous benefits, making it an attractive paradigm for developers. One notable advantage is the ease of testing and debugging. Since functional programs emphasize pure functions that always yield the same output for a given input, side effects are minimized. This predictability simplifies the identification and resolution of bugs, as functions can be tested in isolation.
Improved modularity is another significant benefit. Functional programming promotes the creation of smaller, reusable functions that can be easily combined to build complex systems. This modular approach not only enhances code reusability but also simplifies maintenance and updates. Developers can make changes to individual functions without worrying about ripple effects throughout the system.
Furthermore, functional programming enhances readability. By emphasizing immutability and stateless functions, code is often more straightforward and easier to understand. This clarity is crucial for collaborative projects where multiple developers need to interpret and modify the codebase. Enhanced readability can also facilitate better documentation and reduce onboarding time for new team members.
Better support for concurrent processing is a pivotal advantage of functional programming. Pure functions, being stateless and without side effects, are inherently thread-safe. This characteristic simplifies the development of parallel and distributed systems. For instance, in the realm of big data processing, tools like Apache Spark leverage functional principles to handle large-scale data across multiple nodes efficiently.
Real-world examples highlight the practical benefits of functional programming. For example, financial institutions often adopt functional languages like Haskell to ensure reliability and accuracy in their critical systems. Similarly, companies like Twitter have embraced Scala, a language that combines object-oriented and functional programming, to enhance scalability and maintainability of their codebase.
Common Functional Programming Languages
Functional programming has gained significant traction, encouraging the development and usage of several languages that support or exclusively focus on functional paradigms. Notably, Haskell, Scala, Erlang, and F# stand out among these languages. Each has its history and unique features, which makes them suitable for different use cases.
Haskell
Haskell, named after the logician Haskell Curry, is a statically typed, purely functional programming language introduced in 1990. It emphasizes immutability and lazy evaluation, making it ideal for tasks that require high reliability and performance. Key features include type inference, pattern matching, and higher-order functions.
Here is a simple Haskell function:
square :: Int -> Intsquare x = x * x
Haskell is widely used in academic research and industries requiring robust mathematical computations.
Scala
Scala, short for “scalable language,” was created in 2003, blending object-oriented and functional programming paradigms. It runs on the Java Virtual Machine (JVM), enabling seamless integration with Java libraries. Key features of Scala include its concise syntax, immutable collections, and powerful type system.
A Scala function looks like this:
def square(x: Int): Int = x * x
Scala is popular in big data processing frameworks, such as Apache Spark, and in backend server development.
Erlang
Erlang dates back to 1986, originally developed by Ericsson for telecommunication applications. It excels in building concurrent, distributed systems. Notable features include lightweight process creation, robust error handling, and hot code swapping.
Example of an Erlang function:
square(X) -> X * X.
Erlang is frequently used in real-time systems, such as messaging platforms and telephony applications.
F#
F#, introduced in 2005 and part of the .NET ecosystem, is designed by Microsoft Research as a multi-paradigm language that supports both functional and object-oriented programming. F# boasts type providers for seamless data access, powerful async programming, and succinct syntax.
Example of an F# function:
let square x = x * x
F# is commonly employed in data analytics, financial modeling, and domain-specific languages.
Functional Programming in Mainstream Languages
Functional programming has gained significant traction in the realm of modern software development. While traditionally it was the domain of languages specifically designed for the paradigm, such as Haskell and Lisp, many mainstream programming languages have adopted functional programming principles to leverage their benefits. This section examines how languages such as JavaScript, Python, and Java integrate and apply functional programming concepts.
JavaScript is often recognized for its strong functional programming capabilities. With first-class functions, higher-order functions, and the use of closures, JavaScript encourages functional programming practices. For example, the Array methods such as map
, filter
, and reduce
exemplify functional programming in action. Consider this simple illustration:
const numbers = [1, 2, 3, 4];const doubled = numbers.map(n => n * 2); // [2, 4, 6, 8]
In this snippet, map
is a higher-order function that applies the provided function to each element of the array, returning a new array with the results. Such functional patterns are prevalent in modern JavaScript development, supporting the creation of more predictable and maintainable code.
Python, while primarily an object-oriented language, also embraces functional programming features. Python supports first-class functions and includes standard library functions like map
, filter
, and reduce
. List comprehensions and generator expressions further supplement Python’s functional programming toolbox:
numbers = [1, 2, 3, 4]doubled = list(map(lambda x: x * 2, numbers)) # [2, 4, 6, 8]
This capability allows Python developers to write more declarative and concise code, benefiting from functional programming’s strengths in handling collections and side-effect management.
Java, traditionally known for its imperative and object-oriented capabilities, has also evolved to include functional programming features. The introduction of lambda expressions and the Streams API in Java 8 signified a major shift. By enabling more declarative data processing, these features have driven better abstraction and less boilerplate:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4);List<Integer> doubled = numbers.stream().map(n -> n * 2).collect(Collectors.toList());
The Stream API allows developers to efficiently handle data transformations and aggregations in a functional style, marking a significant advancement in Java’s approach to software development.
These examples illustrate how functional programming principles are enriching mainstream languages, driving more efficient, concise, and maintainable code in modern development practices. By adopting functional paradigms, developers can leverage the best traits of paradigms, enhancing their coding repertoire substantially.
Challenges and Limitations
Adopting functional programming (FP) comes with its share of challenges and limitations. Firstly, there is a steep learning curve for beginners. Functional programming often necessitates a shift in thinking, moving away from the imperative style prevalent in many traditional languages. Concepts such as immutability, pure functions, and higher-order functions can be daunting for those accustomed to stateful programming and side effects. This steep learning curve requires a significant time investment to master, potentially deterring new developers.
Another challenge lies in performance concerns. Functional programming can sometimes lead to less efficient execution. For instance, the extensive use of immutable data structures, while improving code’s predictability, can impact memory and processing speed. Lazy evaluation, a technique used to defer computations until necessary, might introduce overhead and inefficiencies in scenarios requiring immediate computations. Thus, performance can become a hurdle when working in environments where computational speed and memory usage are critical.
Integration with existing imperative codebases also poses significant challenges. Enterprises with large, existing codebases written in imperative or object-oriented paradigms might face difficulties in incorporating functional programming methodologies. The paradigms are fundamentally different, making it challenging to reconcile state-based, mutable code with stateless, immutable FP concepts. This often requires substantial refactoring and redesigns, adding complexity and potential for introducing bugs.
To overcome these challenges, several strategies can be employed. Offering comprehensive training and resources for developers can mitigate the learning curve. Optimizing performance through hybrid approaches, combining functional and imperative styles where necessary, can enhance efficiency. Tools and frameworks designed to bridge the gap between FP and imperative code can facilitate smoother integration. Adaptive techniques, incremental adoption, and utilizing modules or microservices can also ease the transition.
Functional Programming in Real-World Applications
Functional programming (FP) has carved a significant niche for itself across various industries, underscoring its versatility and efficacy. Its principles have been harnessed to tackle complex problems, drive efficiency, and reduce errors in several critical sectors. This section elucidates how functional programming principles are applied in real-world scenarios, with notable examples from finance, telecommunications, and web development.
In the finance industry, accuracy and reliability are paramount. Functional programming thrives in such environments due to its ability to produce deterministic results and manage immutable data structures efficiently. For instance, Jane Street, a renowned proprietary trading firm, employs OCaml, a functional programming language, to execute its trading algorithms. The immutability and strong type system of OCaml enable Jane Street to build robust and error-free applications, an advantage that can spell the difference between profit and loss in high-stakes trading environments.
Telecommunications, another sector that demands high performance and reliability, has also embraced functional programming. Ericsson, a global leader in this field, utilizes Erlang, a language built on functional programming principles, to power its telecommunication systems. Erlang’s concurrency model and fault-tolerant characteristics are particularly suited for the needs of telecommunications, ensuring seamless communication and data transmission across vast networks. By leveraging Erlang’s strengths, Ericsson can maintain and scale its systems effectively while minimizing downtime.
Web development, though a diverse and rapidly evolving domain, also benefits from functional programming. For instance, Facebook uses Haskell in parts of its spam detection systems. Haskell’s capabilities for handling pure functions and higher-order functions make it ideal for creating complex algorithms that can detect and mitigate spam efficiently. Additionally, Airbnb has adopted Scala, another functional programming language, for developing its backend services. Scala’s abstractions and immutable data structures facilitate the creation of scalable and maintainable systems, critical for handling millions of users globally.
These examples clearly illustrate the advantages and practicalities of functional programming in real-world applications. Its principles have been effectively integrated into industry-scaled projects, driving innovation and operational excellence across various sectors.
Future of Functional Programming
Functional programming has been gaining traction across multiple sectors, heralding a promising future characterized by increased adoption, advanced language features, and evolving tools and frameworks. Firstly, industries are recognizing the benefits of functional programming paradigms in achieving more robust, maintainable, and scalable codebases. The heightened emphasis on software reliability and error handling makes functional programming an appealing choice, especially in sectors like finance, healthcare, and cybersecurity.
The evolution of functional programming languages such as Haskell, Scala, and F# also plays a pivotal role. These languages are continuously being enhanced with new features that simplify functional concepts for developers, thereby lowering the barrier to entry. Innovations such as improved type systems, better tooling, and more extensive libraries contribute to making functional programming more accessible and practical for diverse applications.
Concurrently, the ecosystem surrounding functional programming is rapidly maturing. Development tools and frameworks are evolving to support functional paradigms more robustly. Enhanced performance profiling tools, sophisticated integrated development environments (IDEs), and powerful debugging utilities are essential factors enabling smoother development workflows. Moreover, the increasing incorporation of functional programming concepts into mainstream languages like JavaScript and Python signifies a broader acceptance and blending of functional paradigms with imperative programming, catering to a wider developer base.
One notable trend is the growing interest in immutability and pure functions within the software engineering community. These principles are particularly effective in developing concurrent and parallel applications, addressing the complexities of multi-core processing and distributed computing environments. As enterprises strive for more efficient, error-free, and scalable applications, the demand for functional programming skills is expected to rise.
In summary, the future of functional programming looks promising, marked by its increasing adoption across various industries, ongoing improvements in language features, and the continuous evolution of supporting tools and frameworks. This trajectory signifies not just a transient trend but a profound shift towards more reliable and maintainable software development practices.