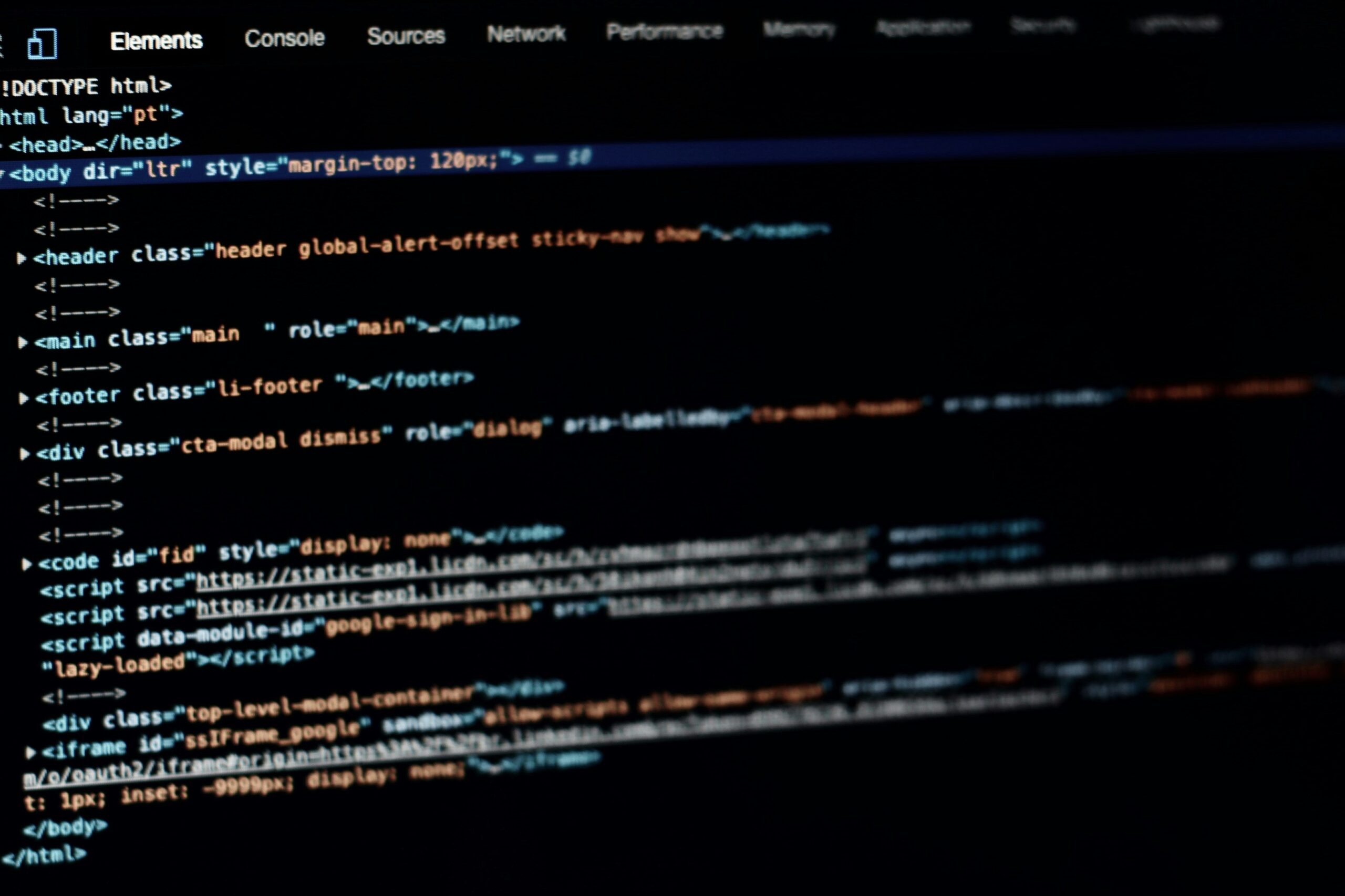
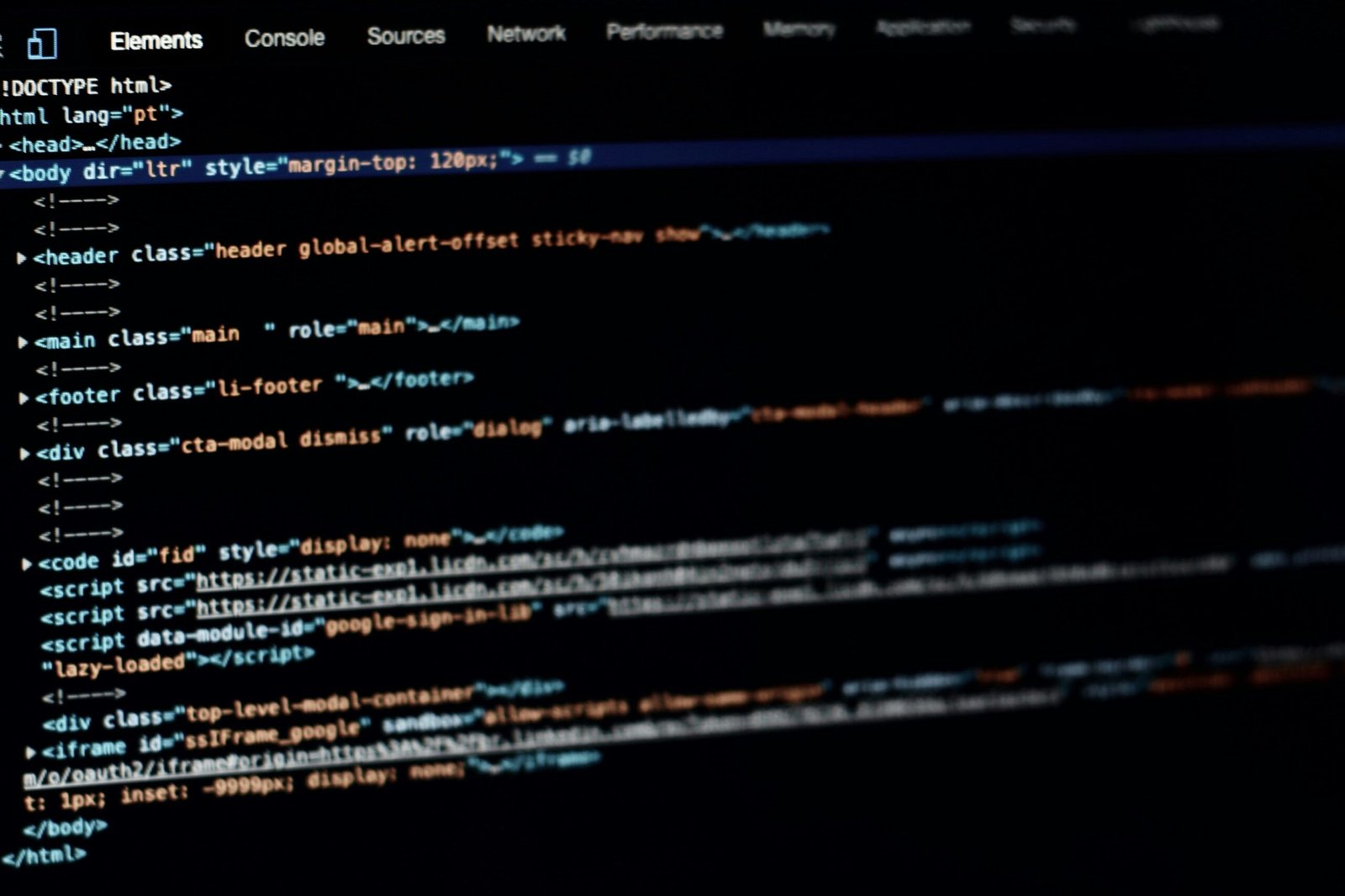
Introduction to Lisp
Lisp, an acronym for “LISt Processing,” stands as one of the earliest high-level programming languages, laying the groundwork for many concepts we see in contemporary programming today. Developed in 1958 by John McCarthy while he was at the Massachusetts Institute of Technology (MIT), Lisp has had a profound impact on the field of computer science and artificial intelligence.
John McCarthy’s vision for Lisp emerged from his pioneering work in symbolic computation and recursive function theory. He sought a system that could handle symbolic expressions as data, unlike the primarily numerical computations that were the focus at that time. This visionary approach led to the development of Lisp’s unique features, such as its use of parenthetical notation, symbolic expressions, and dynamic typing.
Since its inception, Lisp has been instrumental in numerous academic and practical advancements. It introduced fundamental programming constructs like tree data structures, automatic storage management, and higher-order functions, many of which have influenced the design of modern programming languages. Lisp’s homoiconicity—where the code and data have the same structure—has also allowed for powerful metaprogramming capabilities, making it a favorite among researchers for developing domain-specific languages and prototypes.
Over the decades, various dialects of Lisp have emerged, including Common Lisp, Scheme, and Clojure, each extending its core concepts for different applications. Despite the emergence of newer languages, Lisp’s timeless principles continue to underpin major developments in fields such as artificial intelligence, algorithm research, and software engineering. Its resilience and adaptability highlight its significance and enduring influence in the world of computing.
Key Features and Characteristics of Lisp
Lisp distinguishes itself in the landscape of programming languages through several unique features that contribute to its versatility and expressiveness. One of the defining characteristics of Lisp is the use of S-expressions (Symbolic Expressions) for both code and data. This dual use of S-expressions underpins the language’s syntactical uniformity, enabling seamless manipulation and transformation of code as data. This attribute not only simplifies the parsing process but also enhances the language’s metaprogramming capabilities.
Another significant feature is Lisp’s use of prefix notation, also known as Polish notation. In this notation, operators precede their operands, which stands in contrast to infix notation more commonly seen in languages like C or Java. For example, to add two numbers in Lisp, one would write (+ 2 3)
instead of 2 + 3
. This consistent structure eliminates the need for operator precedence rules and parentheses for grouping in complex expressions, further simplifying the process of creating and understanding programs.
Lisp’s powerful macro system is another cornerstone of its expressiveness. Unlike macros in simpler languages, Lisp macros operate at the syntactic level, allowing developers to effectively extend the language in ways that are syntactically indistinguishable from the core language constructs. This ability to mold the language to specific problems is exemplified by the creation of new syntactic constructs and domain-specific languages within Lisp itself.
Furthermore, Lisp places a strong emphasis on recursive functions, leveraging the natural fit of recursion with its syntactic structure and functional programming paradigm. Recursive functions are a natural way to approach problems such as traversing tree structures or parsing nested lists, which are common in Lisp programming. This emphasis on recursion allows developers to write more elegant and concise code, enhancing readability and maintainability.
The Lisp Family: Dialects and Variants
Lisp, one of the oldest high-level programming languages, has evolved into a family of dialects with unique characteristics. Each dialect has adapted to different needs and disciplines, leading to the emergence of Common Lisp, Scheme, and Clojure as some of the most popular variants. Understanding their differences and similarities offers insight into their specific applications and communities.
Common Lisp stands as one of the most versatile and comprehensive dialects in the Lisp family. It was designed to be a standard, unifying the various earlier Lisp dialects. Its rich set of features, including dynamic typing, garbage collection, and a powerful macro system, make it suitable for a wide range of applications, from artificial intelligence to rapid prototyping. The Common Lisp community appreciates its robustness and extensive libraries, which facilitate the development of complex programs.
Scheme, another prominent dialect, takes a minimalist approach compared to Common Lisp. It emphasizes simplicity and elegance, featuring a smaller standard library and cleaner semantics. Scheme’s minimalistic design makes it a favorite for educational purposes and research in computer science, where clarity and precision are paramount. The dialect’s conciseness and ease of use have also made it popular in academic settings, where it serves as an ideal language for teaching fundamental programming concepts.
Clojure, a modern dialect of Lisp, integrates seamlessly with the Java Virtual Machine (JVM), making it compatible with a vast ecosystem of Java libraries. It introduces immutability and a strong focus on concurrency to harness the full potential of multi-core processors. Clojure’s emphasis on functional programming and its ability to handle state changes in a controlled manner have earned it a significant following in the software development community, particularly among those building scalable, concurrent systems.
Despite their differences, these Lisp dialects share core principles such as code-as-data (homoiconicity), powerful macro systems, and a basis in symbolic computation. Each has cultivated a dedicated community, contributing to forums, developing libraries, and organizing events. Whether it’s Common Lisp’s extensive feature set, Scheme’s minimalistic purity, or Clojure’s modern concurrency capabilities, each dialect offers unique advantages tailored to specific use cases.
Functional Programming in Lisp
Functional programming is a core aspect of Lisp, embodying concepts such as first-class functions, higher-order functions, and immutability. Functional programming in Lisp promotes a declarative approach to coding, focusing on what should be computed rather than how to compute it.
First-class functions in Lisp mean that functions are treated as any other data type. They can be assigned to variables, passed as arguments, and returned from other functions. This characteristic allows for a high degree of modularity and reusability in Lisp programs, enabling developers to build more flexible and expressive code. For example, the `lambda
` function in Lisp is used to create anonymous functions, which can subsequently be used as arguments to other functions.
Higher-order functions take this concept a step further. These are functions that can accept other functions as parameters or return them as results. In Lisp, higher-order functions are extensively used to simplify operations on lists and other collections. Common higher-order functions include `mapcar
` and `reduce
`. For example, `mapcar
` applies a given function to each element of a list and returns a new list of the results, encouraging concise and readable code.
Immutability is another foundational principle in functional programming with Lisp. Immutable data structures ensure that the data cannot be modified after creation, leading to more predictable and bug-free code. Lisp’s native support for lists and its powerful macro system both leverage immutability to help developers build robust applications. By emphasizing immutability, Lisp encourages a programming style where variables are never reassigned, enhancing clarity and reliability.
One of the prime examples of functional programming in Lisp is the use of recursion. Recursion replaces traditional loops, providing an elegant way to solve problems by defining a function in terms of itself. This further enhances the declarative nature of Lisp code, making it easier to reason about the operations being performed.
Overall, functional programming in Lisp fosters a robust, modular, and expressive coding environment. The principles of first-class functions, higher-order functions, and immutability significantly contribute to Lisp’s reputation as a powerful tool for complex problem-solving.
Lisp’s Influence on Modern Programming Languages
The Lisp programming language, originally developed in the late 1950s, has had a profound influence on the design and development of various modern programming languages. One of Lisp’s most notable contributions is the introduction of first-class functions, a concept that has been widely integrated into contemporary languages. For instance, JavaScript heavily relies on this concept, allowing functions to be stored in variables, passed as arguments, and returned from other functions. This feature is pivotal in enabling JavaScript’s flexibility and supports the development of powerful frameworks and libraries.
Another significant area where Lisp’s impact is evident is in the realm of list processing and manipulation. Python, a language well-known for its readability and simplicity, has adopted list comprehensions, a feature highly reminiscent of Lisp’s emphasis on list manipulation. List comprehensions in Python provide a compact and expressive way to create and manipulate lists, showcasing Lisp’s lasting legacy in influencing efficient data handling techniques.
Lisp’s macro system has also left an indelible mark on modern metaprogramming techniques. Macros in Lisp enable developers to freely manipulate code as data and create domain-specific languages, granting unprecedented flexibility and expressive power. This concept has inspired advanced metaprogramming capabilities in languages like Rust and Julia. In Rust, macros empower developers to write complex and reusable code more easily, leading to more efficient and maintainable systems. Julia’s metaprogramming allows users to generate and include code dynamically, optimizing performance and extending the language’s capabilities.
Furthermore, the core principles of functional programming embodied in Lisp have found their way into languages such as Haskell and Scala. These languages embrace immutability, higher-order functions, and expressive type systems, all of which are hallmarks of Lisp’s philosophy. Elements like lambda expressions, anonymous functions, and recursive programming found in many modern languages also trace their roots back to Lisp.
In sum, the foundational concepts introduced by Lisp continue to shape the landscape of modern programming, underpinning the development of versatile and powerful programming languages. Whether through first-class functions, advanced list handling, or metaprogramming, Lisp’s influence remains deeply embedded in the evolution of software development.
Practical Applications of Lisp
Lisp, arcane yet versatile, has found its stronghold in numerous realms across the technological landscape. One of the most prominent applications of Lisp is in artificial intelligence (AI) research, where its unparalleled capability for symbolic computation and manipulation of complex data structures makes it indispensable. Languages like Common Lisp and Scheme have laid the groundwork for pioneering AI developments. For instance, the expert system DART (Dynamic Analysis and Replanning Tool), which significantly optimized logistics planning for the U.S. military during Operation Desert Storm, was developed using Lisp.
Beyond AI, Lisp excels in the domain of rapid prototyping. Its dynamic typing, coupled with the ability to support high-level abstractions, enables developers to rapidly conceive and iterate on prototypes. This flexibility is particularly beneficial in innovative environments where time and adaptability are paramount. Additionally, Lisp’s macro system, which allows developers to redefine the syntax and semantics of the language, underscores its adaptability in crafting domain-specific languages tailored for unique project requirements.
In academic settings, Lisp serves as an instrumental tool in computer science education and research. Its unique syntax, based on list processing, provides an ideal framework for teaching fundamental concepts of recursion, lambda calculus, and functional programming. Lisp’s influence extends deeply into theoretical computer science, where it has shaped curricula and research paradigms for generations of computer scientists. Scholars worldwide use Lisp to explore computational theory, language design, and algorithm development.
Numerous notable projects and systems reflect the practical utility of Lisp. Emacs, one of the most powerful and extensible text editors, is built on Emacs Lisp. This integration allows users to tailor nearly every aspect of the editor to their liking, highlighting Lisp’s extensibility. Another exemplary case is Maxima, a computer algebra system, which leverages Lisp for symbolic computation, enabling complex mathematical operations.
From AI to rapid prototyping to academic research, the use of Lisp programming language underscores its versatility and enduring significance in both practical applications and theoretical explorations.
Learning Lisp: Resources and Community
Learning Lisp can be greatly facilitated with the right resources. For those new to the language, numerous books, online tutorials, and courses provide a solid foundation. One of the most acclaimed books is “Structure and Interpretation of Computer Programs” by Harold Abelson and Gerald Jay Sussman. This book is often praised for its thorough explanation of Lisp concepts and is widely used in academic settings. “Practical Common Lisp” by Peter Seibel is another excellent resource, known for its practical approach to teaching the language, making it ideal for self-learners.
Online courses and tutorials offer a flexible and interactive learning experience. Websites like Coursera, edX, and Udacity offer courses that cover everything from basic syntax to advanced Lisp programming techniques. Additionally, platforms like Codecademy provide interactive exercises that can help learners master the fundamental aspects of Lisp programming. For a more hands-on approach, the website learnXinyminutes.com provides a concise overview suitable for quick learning sessions.
The Lisp community is vibrant and welcoming, offering numerous forums and user groups where enthusiasts can connect. Websites such as Reddit’s r/lisp and the Lisp tag on Stack Overflow serve as active hubs for discussion, troubleshooting, and sharing knowledge. Furthermore, mailing lists like the “comp.lang.lisp” offer an excellent archive of discussions and are a testament to the language’s ongoing development and support.
Lisp conferences and meetups also provide opportunities for professional networking and learning. The International Lisp Conference (ILC) is one such event where experts present their latest research and innovations in the language. Local user groups, such as the LispNYC and the European Lisp Symposium, also play a crucial role by organizing regular meetups, hackathons, and talks that foster community engagement and knowledge sharing.
By leveraging these resources and participating in the vibrant Lisp community, learners can deepen their understanding of the language and contribute to its evolution.
Future of Lisp in the Software Industry
The trajectory of Lisp in the technology landscape remains promising, characterized by its enduring adaptability and flexibility. While Lisp may not enjoy the widespread popularity of more contemporary programming languages, ongoing efforts to modernize and extend its capabilities suggest a bright future. The continuous development of new dialects, such as Clojure and Racket, demonstrate the language’s capacity to evolve and integrate innovative features, ensuring relevance in modern software development.
One significant area where Lisp shows tremendous potential is in the burgeoning fields of machine learning and data science. Its symbolic computing capabilities make it particularly suited for the development of intelligent algorithms. The capability to process symbolic information efficiently positions Lisp as a robust candidate for implementing machine learning models, especially those requiring symbolic manipulation and reasoning. Ongoing research and experimental projects continue to explore this synergy, underlining Lisp’s potential contribution to these cutting-edge domains.
Furthermore, the rise of artificial intelligence research has prompted a renewed interest in languages that can handle complex computations with clarity and precision. Lisp, with its elegant syntax and powerful macro system, offers a substantial advantage in prototyping and developing AI systems. As industries increasingly shift toward automation and intelligent systems, Lisp’s inherent strengths in handling sophisticated algorithms and recursive processes will likely see a resurgence in utility and application.
Innovative applications of Lisp in modern computing are not limited to AI and data science. The language’s influence extends into fields like bioinformatics, financial modeling, and even hardware design through domain-specific languages. These diverse applications highlight Lisp’s versatility and the role it can play in solving domain-specific problems, further cementing its value in the software industry.
Despite the emergence of numerous programming languages tailored for specific tasks, Lisp’s unique characteristics and its ability to adapt to changing technological landscapes make it a timeless tool for sophisticated software solutions. As the tech industry continues to evolve, so too will the opportunities for Lisp to leave an indelible mark on future innovations.