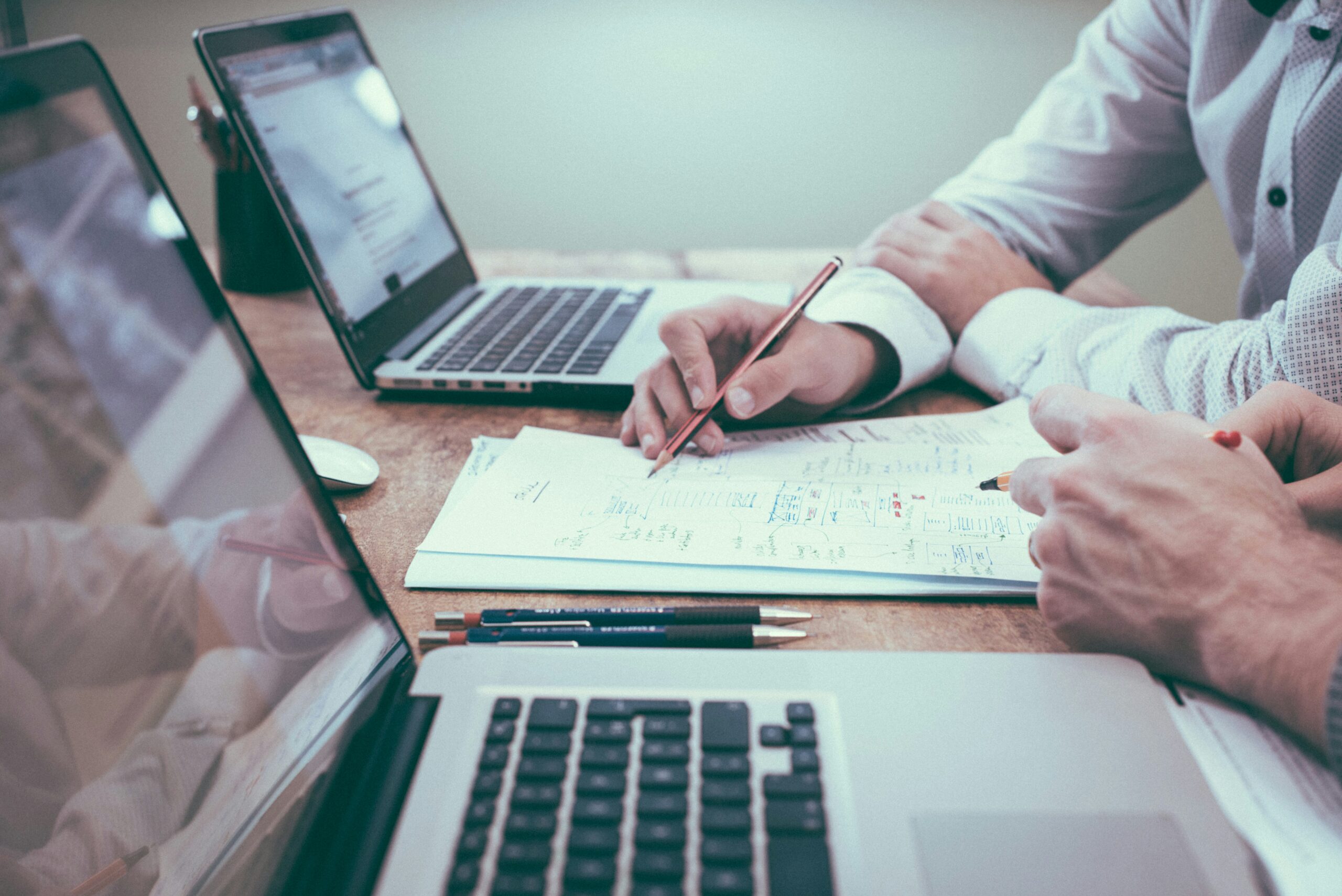
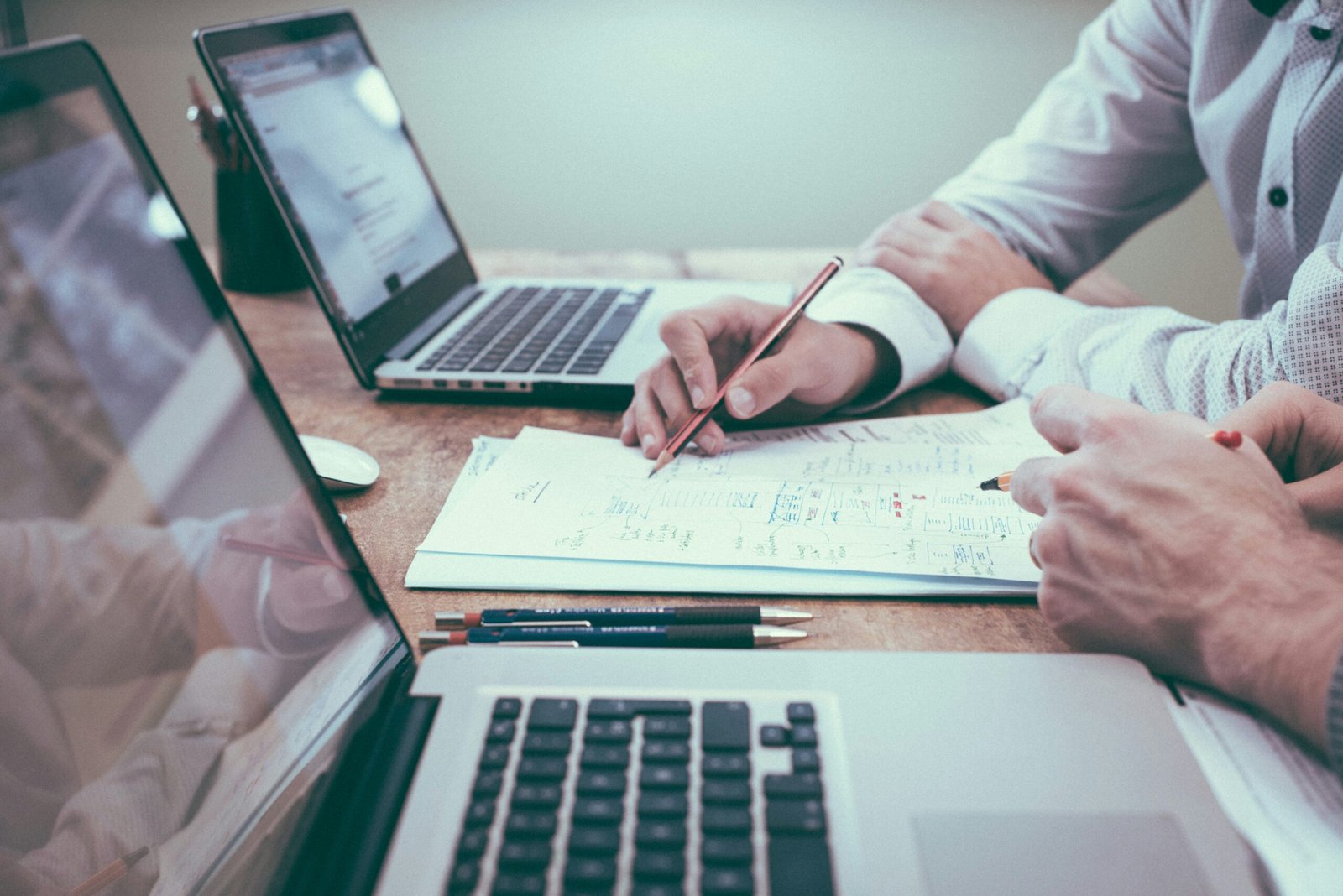
Introduction to Programming Paradigms
Programming paradigms are fundamental styles or approaches to programming that embody particular philosophies and techniques. Understanding programming paradigms is crucial for grasping the diverse methodologies that exist within the field of computer science. These paradigms dictate how programmers write and structure code, influencing the design and functionality of software applications.
A programming paradigm can be considered a pattern or a model that guides how problems are solved and how various tasks are performed within a programming environment. Over the decades, as technology has advanced and programming needs have evolved, various paradigms have emerged to address distinct challenges and to optimize certain processes. The evolution of programming paradigms reflects the continual search for more effective, efficient, and scalable ways to develop software.
Early programming paradigms, such as procedural programming, were primarily focused on sequence and control structures. As complexity in software systems grew, the need for more sophisticated approaches led to the development of other paradigms like object-oriented programming, which encapsulates data and behavior into objects, and functional programming, which emphasizes immutability and pure functions. Newer paradigms such as parallel and reactive programming have further expanded the toolkit available to developers, aiming to handle modern computational needs like concurrency and real-time data streams.
The existence of different programming paradigms allows developers to choose the most appropriate tools and techniques for the specific problems they face. For instance, while one paradigm might be suitable for building straightforward, linear logic systems, another might excel in handling events and state changes. By studying and understanding various paradigms, programmers are better equipped to select the optimal approach for their projects. Consequently, familiarity with multiple programming paradigms vastly enhances a programmer’s adaptability and problem-solving skills.
Procedural Programming
Procedural programming stands as one of the foundational paradigms in the field of computer science. This paradigm revolves around the notion of procedure calls, where code is organized into blocks called procedures or routines. Each procedure performs a specific task and can be reused throughout the program, promoting modularity and organization. By employing a step-by-step approach to problem-solving, procedural programming advocates for a linear sequence of instructions that are executed in a specific order.
Among the key characteristics of procedural programming are its emphasis on sequential execution, use of global and local variables, and reliance on procedures for task execution. These features ensure that code is not only easier to write but also more accessible to debug and maintain. One hallmark of this paradigm is its straightforward and methodical approach to tackling problems, making it particularly suitable for beginners.
Several prominent programming languages epitomize procedural programming. C, for instance, is a highly influential language known for its performance and close alignment with hardware. It has been instrumental in developing operating systems, embedded systems, and various software applications. Pascal, another notable procedural language, was designed with teaching in mind and has been acclaimed for its structured approach and clarity. Both languages have played pivotal roles in shaping modern software development.
Procedural programming is especially effective in scenarios that benefit from a clear and linear sequence of operations. It is well-suited for algorithm implementation, file manipulation tasks, and computational processes that require precise control over the order of execution. Moreover, its simplicity and clarity make it an excellent choice for educational purposes, providing learners with a solid foundation in programming concepts.
Given its structured approach and emphasis on order and simplicity, procedural programming remains a vital paradigm, particularly in environments where predictability and ease of debugging are paramount. Its principles continue to influence modern programming practices, underscoring the enduring significance of procedural techniques in software development.
Object-Oriented Programming (OOP)
Object-Oriented Programming (OOP) is a programming paradigm that emphasizes the use of objects and classes. This paradigm is built around the concept of encapsulating data and behavior into single units known as objects. Objects are instances of classes, which define a blueprint for the object’s structure and behavior. Key concepts in OOP include encapsulation, inheritance, and polymorphism.
Encapsulation involves bundling the data (attributes) and methods (functions that operate on the data) within a class and restricting access to some of the object’s components. This creates a clear structure, making the code more modular and easier to manage. For instance, private variables can only be accessed through public methods, which helps in maintaining control over data integrity.
Inheritance allows a class to inherit properties and methods from another class, promoting code reusability. It facilitates the creation of hierarchical classifications and enables developers to extend functionality without modifying existing code. Polymorphism, on the other hand, enables the ability to call the same method on different objects, allowing for one interface to be used for a general class of actions. This is achieved through method overriding and overloading.
Illustrative examples of popular OOP languages include Java, C++, and Python. Java is widely used for building enterprise-level applications due to its robustness and portability. C++ offers powerful performance, making it ideal for system/software development and game programming. Python is known for its simplicity and versatility, finding applications across fields such as web development, data analysis, and scientific computing.
The advantages of OOP are numerous. Its emphasis on code reusability through inheritance and class modularity enhances scalability and reduces redundancy. Encapsulation ensures data integrity and security, while polymorphism contributes to flexible and maintainable code. Common real-world applications of OOP include large-scale software applications, web frameworks, and mobile app development, among others. These attributes make OOP a preferred choice for many developers seeking organized and efficient programming solutions.
Functional Programming
Functional programming (FP) is a programming paradigm that emphasizes the use of mathematical functions and immutability. This paradigm integrates higher-order functions, first-class functions, and pure functions to create more predictable and testable code. Higher-order functions are functions that can take other functions as arguments or return them, facilitating functional composition and abstraction. First-class functions treat functions as first-class citizens, meaning they can be passed around as values. Pure functions, devoid of side effects, ensure that the output is consistent given the same inputs.
Notable functional programming languages include Haskell, Lisp, and Scala. Haskell is a purely functional language, meaning it adheres strictly to the principles of the paradigm. Lisp, known for its symbolic expression and code-as-data philosophy, offers robust functional programming features. Scala, integrating both object-oriented and functional programming, provides a flexible language framework, making it popular in enterprise environments.
Functional programming offers significant benefits, including ease of testing and parallelism. Pure functions, by nature, are easier to test because they do not rely on external state, ensuring that tests yield predictable results. The immutability aspect makes state management more straightforward and reduces the chances of bugs due to unwanted side effects. Additionally, the paradigm’s emphasis on immutability and statelessness facilitates parallelism, as functions can be executed concurrently without risk of state corruption.
Scenarios where functional programming proves particularly advantageous include data transformation processes, real-time systems, and applications requiring high reliability. For instance, in data processing pipelines, functional programming’s declarative style enables clear and concise transformations. Real-time systems benefit from the predictability and testability of pure functions, and applications demanding high reliability leverage the reduced risk of side effects for increased robustness.
Logic Programming
Logic programming is a paradigm grounded in formal logic, fundamentally differing from imperative or declarative approaches. At its core, logic programming involves expressing facts and rules about problems in a form of logical statements, from which conclusions are derived using rigorous logical inference methods. It is an effective methodology for domains where intricate relationships and conditions are quintessential, including artificial intelligence, expert systems, and natural language processing.
The principles of logic programming stem from the logical relation between facts and rules. Facts represent basic assertions about the world, while rules delineate how new facts can be derived from existing ones. For instance, if it is a fact that “All humans are mortal,” and another fact that “Socrates is a human,” we can infer by a rule that “Socrates is mortal.” This logical inference forms the bedrock of logic programming, allowing complex problem-solving through systematic deduction.
Prolog, short for “Programming in Logic,” stands as the quintessential logic programming language. Prolog’s syntax and operational semantics are engineered to naturally facilitate logical relations and automate logical deductions. Users define a set of facts and rules, and Prolog’s inference engine processes these to infer conclusions. Prolog’s efficiency in handling symbolic representations and logical relations has cemented its niche in various domains. Specifically, Prolog is widely implemented in fields such as artificial intelligence—enabling the development of intelligent systems that can reason, learn, and provide solutions.
Expert systems—a branch of AI—use logic programming to replicate the decision-making ability of a human expert. These systems utilize facts and inference rules to process inputs and generate expert-level advice or decisions. Furthermore, in natural language processing, logic programming plays a crucial role in understanding and generating human language, where the structured definition of grammatical rules and logical inference can manipulate and interpret complex linguistic data.
In summary, logic programming presents a robust paradigm for tackling problems requiring sophisticated logical reasoning and precise inference, with Prolog remaining an exemplary tool for developers in specialized computational domains.
Scripting Paradigms
Scripting paradigms are a crucial part of the programming landscape, especially when dealing with high-level scripts that require swift and efficient development. Unlike compiled languages, scripting languages such as Python, Ruby, and JavaScript offer significant ease of use and faster development cycles. This ease of use is primarily due to their interpretive nature, which eliminates the need for explicit compilation steps.
Python, for instance, is renowned for its readability and comprehensive standard library, making it an excellent choice for both beginners and seasoned developers. Ruby’s simplicity and elegant syntax empower developers to write concise and readable code. JavaScript, with its omnipresence in web development, facilitates client-side and server-side scripting, thus enabling dynamic and interactive web applications.
The flexibility and versatility of scripting paradigms make them ideal for a variety of applications. Automation is a common use case, wherein repetitive tasks can be streamlined through scripting, significantly enhancing productivity. Scripting languages excel in web development; for example, JavaScript is pivotal in front-end development, while server-side scripting can be effectively managed by languages like Python and Ruby via frameworks like Django and Ruby on Rails, respectively. Additionally, scripting paradigms are prevalent in rapid prototyping, allowing developers to quickly iterate and validate concepts without the overhead of more rigid programming languages.
Overall, the scripting paradigm offers a dynamic and scalable solution for various programming needs. Its inherent ease of use, coupled with the reduced development time, makes it a preferred choice for numerous applications, fostering innovation and efficient problem-solving in modern software development.
Declarative Programming
Declarative programming is a paradigm that emphasizes the logic of computation without explicitly delineating its control flow. Unlike imperative programming, which focuses on the ‘how’ by specifying the steps and processes to achieve a solution, declarative programming concentrates on the ‘what’ by defining the desired outcome. This approach simplifies the development process by abstracting the underlying mechanics and allowing languages or frameworks to manage the operational details.
Notable examples of declarative programming languages and frameworks include SQL for database management, and HTML/CSS for web layout design. SQL (Structured Query Language) allows users to efficiently query and manage database systems by stating what data to retrieve or manipulate, leaving the database engine to determine the most efficient strategy to execute these queries. Similarly, in web design, HTML (Hypertext Markup Language) and CSS (Cascading Style Sheets) enable developers to describe the structure and appearance of web pages respectively, focusing on the final visual presentation rather than the procedural steps to render it.
Declarative programming finds extensive applications beyond databases and web design, extending into areas like UI (User Interface) design and configuration management. In UI design, frameworks like React and Vue.js leverage a declarative style to simplify user interface creation and modification, enhancing productivity and maintainability. In configuration management, tools such as Ansible and Puppet adopt declarative paradigms to define system configuration states, thus facilitating system automation and ensuring consistency across environments.
By focusing on the ‘what,’ declarative programming improves readability and reduces complexity, making it suitable for applications where the clarity of the final objective is paramount. It empowers developers to achieve more with less code, streamlining the development process, and fostering more maintainable and scalable solutions.
Multi-paradigm programming is a sophisticated approach in software development that enables developers to harness the strengths of various programming paradigms within a single language. This concept is particularly beneficial in the realm of modern programming, where flexibility and robustness are paramount. Languages such as Python, JavaScript, and C++ exemplify multi-paradigm programming by supporting multiple paradigms, including procedural, object-oriented, and functional programming.
One of the primary advantages of using multi-paradigm languages is the ability to select the most suitable paradigm for a specific task, thereby optimizing code efficiency and readability. For instance, in Python, developers can write procedural code for simple scripts, employ object-oriented techniques for creating complex data structures, and utilize functional programming concepts for handling data transformations. This adaptability makes Python an exemplary tool for both rapid prototyping and large-scale application development.
Similarly, JavaScript, a cornerstone of web development, demonstrates multi-paradigm capabilities by mixuring functional programming features with object-oriented programming. This enables web developers to build highly interactive and sophisticated web applications. JavaScript’s flexibility allows developers to use a functional approach for managing state changes or leverage object-oriented principles to model complex entities, thus crafting more maintainable and scalable codebases.
C++ is another noteworthy example, combining low-level memory manipulation with high-level abstraction mechanisms. This versatility is particularly valuable in system software, game development, and applications requiring significant performance optimization. By integrating procedural, object-oriented, and generic programming paradigms, C++ empowers programmers to write efficient and adaptable code, tailored to diverse requirements.
The ability to merge different paradigms within a single language framework leads to the creation of more flexible software solutions. This flexibility not only enhances code quality but also streamlines the development process, allowing programmers to utilize the most effective approach for a given problem. Consequently, multi-paradigm programming fosters innovation and resilience in software development, equipping developers with the tools needed to navigate an ever-evolving technological landscape.