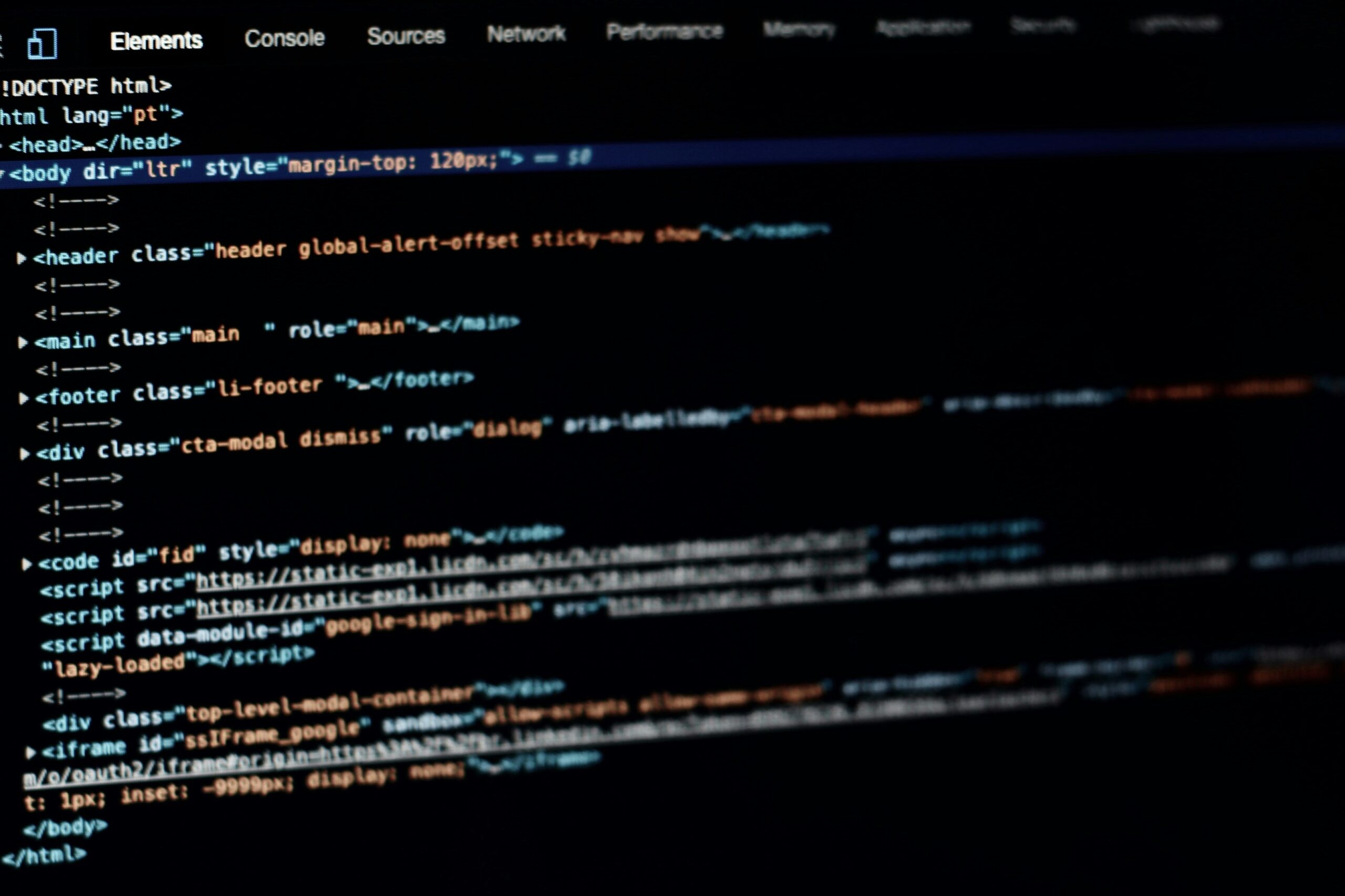
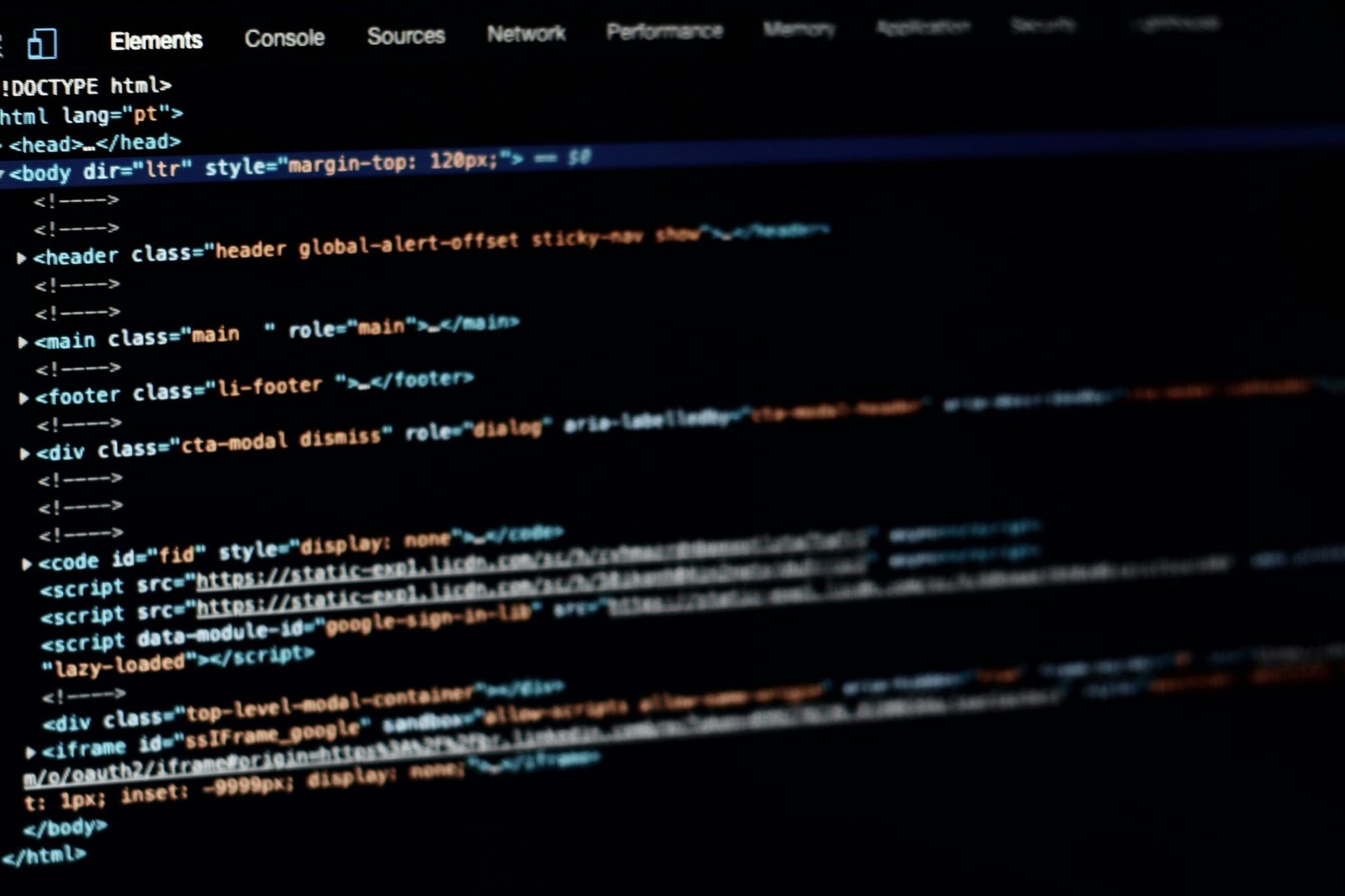
History and Evolution of C
The C programming language, one of the most enduring and widely adopted languages in computing history, originated in the early 1970s at Bell Laboratories. Dennis Ritchie, alongside his colleague Brian Kernighan, developed C from an earlier language known as B, which was itself derived from BCPL (Basic Combined Programming Language). The primary impetus for creating C was to enhance system programming capabilities within the constraints of an evolving computing environment.
During the late 1960s and early 1970s, computing was characterized by the development of operating systems that needed to be efficient and robust. This context led to the creation of the Unix operating system, an ambitious project seeking a platform that facilitated ease of use and effectiveness in system design. The evolution of C was closely intertwined with Unix, as the language was instrumental in crafting this groundbreaking operating system. By 1973, Unix had been entirely rewritten in C, demonstrating the latter’s prowess in delivering portability and efficiency at a time when assembly language had been the norm.
The development and adoption of C did not halt with Unix. Recognizing the need for a standardized version of the language to ensure consistency and portability across different computing systems, the American National Standards Institute (ANSI) formed a committee in 1983. The result of this effort was the ANSI C standard, completed in 1989, which codified the syntax, semantics, and library functions of C. This standard provided a foundational reference that facilitated widespread adoption and adaptation of C in diverse computing applications.
C’s evolution over the decades has also seen significant milestones including the creation of influential languages like C++ and Objective-C, which built upon its principles. Its continued relevance, especially in system programming, embedded systems, and resource-constrained environments, underscores its profound impact on the broader software development landscape. The historical journey from its humble beginnings at Bell Labs to becoming a standardized, foundational language echoes C’s significant role in shaping modern computing.
Core Features and Syntax of C
The C programming language, renowned for its robustness, provides a solid foundation in software development owing to its fundamental features and syntax. The simplicity and efficiency of C start with its data types, which include primitive data types like int, char, float, and more complex structures such as arrays, pointers, and user-defined types like structs and unions. These types help in representing both simple and complex data with clarity and precision.
Another integral aspect of C is its operators. The language supports arithmetic, relational, logical, bitwise, and assignment operators, allowing a wide range of arithmetic and logical operations to be performed succinctly. This often provides a clearer and more direct manipulation of data compared to some high-level languages.
Control structures in C, including if-else conditions, switch statements, and loops like for, while, and do-while, form the backbone of program execution flow. These constructs enable developers to dictate the logical progression of their programs, making decision-making and repetitive tasks streamlined and more manageable.
Functions in C are another cornerstone of its design. C allows the creation of user-defined functions, promoting modularity and code reuse. These functions can return values and accept parameters, fostering a structured approach to coding. The ability to pass functions as parameters to other functions also aligns with its versatile and functional character.
One of the distinctive strengths of C is its emphasis on pointers and manual memory management. Pointers offer powerful capabilities such as dynamic memory allocation, array manipulation, and the creation of complex data structures like linked lists and trees. Manual memory management grants developers control over memory usage, optimizing performance crucially in system-level programming and embedded systems.
Overall, C’s blend of simplicity, efficiency, and control over system resources sets it apart. Its syntax is straightforward yet potent, designed to closely map with machine instructions, ensuring speed and performance. This makes C not only a foundational language for many other languages but also a preferred choice for systems programming, embedded systems, and performance-critical applications.
Standard Libraries and Functions
The ANSI C standard provides a robust collection of standard libraries and functions, which are crucial for both novice and experienced developers. These libraries simplify a wide array of tasks, thereby enhancing productivity and promoting code reuse. One of the fundamental libraries included in the ANSI C standard is the standard input/output library, accessible via #include <stdio.h>
. This library offers essential functions like printf
and scanf
for formatted output and input, thereby facilitating effective communication between programs and their users.
Another significant component is the string handling library, encapsulated in #include <string.h>
. This library is indispensable due to its array of functions for manipulating strings. Functions like strlen
for determining string length, strcpy
for string copying, and strcat
for concatenation, enable programmers to perform complex string operations efficiently.
For mathematical computations, the math.h
library stands as a quintessential tool. It includes a variety of standard mathematical functions, such as sqrt
for square roots, pow
for exponentiation, and sin
, cos
for trigonometric functions. These functions are optimized for performance and accuracy, making them invaluable in scientific computing and engineering applications.
The standard library also addresses memory management through the stdlib.h
library. Functions like malloc
for dynamic memory allocation, calloc
for allocating and zero-initializing arrays, and free
for releasing allocated memory, provide programmers with the necessary tools to handle memory efficiently. Proper use of these functions is essential for developing robust and high-performance applications.
Overall, the standard libraries and functions provided by ANSI C are foundational competencies that streamline many common programming challenges. By offering a well-defined set of utilities for input/output operations, string manipulation, mathematical calculations, and memory management, these libraries not only simplify the development process but also ensure that codebases remain consistent and maintainable across different projects.
Advanced Concepts and Techniques
The C programming language is renowned for its efficiency and control, both of which are enhanced by mastering advanced concepts. One such technique is dynamic memory allocation, which enables the programmer to manage memory manually via functions like malloc
, calloc
, realloc
, and free
. This practice is crucial for scenarios where the amount of data may not be known upfront, such as when processing user input or handling large data sets dynamically.
Structures and unions are another pillar of C’s advanced functionality. A structure (struct
) allows the grouping of variables of different types under a single name, facilitating more coherent data management. For instance, a struct
may represent a student record with fields for name, age, and grades. On the other hand, a union facilitates memory-efficient management of variables by allowing different data types to occupy the same memory space, albeit with restrictions on concurrent use.
Bit manipulation is another area where C shines, offering powerful techniques for handling operations at the bit level. Operations such as bit masking, shifting, and setting can be performed using bitwise operators like &
(AND), |
(OR), ^
(XOR), and ~
(NOT). These techniques are especially beneficial in embedded systems and applications requiring precise control over hardware, such as device drivers and real-time systems.
File handling in C is indispensable for developing applications that read from or write to data stores. Utilizing functions from the stdio.h
library, such as fopen
, fread
, fwrite
, and fclose
, developers can efficiently manage data input and output with external files. This capability is pivotal in applications ranging from simple data logging to complex database management systems.
By integrating these advanced concepts, C programmers can build software that is not only high-performing but also incredibly efficient. Examples include dynamic data structures like linked lists and hash tables, which leverage dynamic memory allocation and structures. Similarly, bit manipulation is widely used in cryptographic algorithms and network protocols. File handling is essential for systems that rely on persistent storage, such as configuration files or database interactions.
C in Systems Programming
The C programming language is renowned for its pivotal role in systems programming. Its influence can be seen across various domains, from operating system development and embedded systems to hardware programming. The distinctive feature that positions C as the language of choice in these areas is its low-level capabilities, which facilitate direct memory access and hardware manipulation.
Operating systems serve as a prime example of C’s utility in systems programming. Renowned operating systems like UNIX, Linux, and Windows have been largely written in C. The primary reason lies in C’s efficiency and control over system resources. By allowing direct interaction with the hardware, developers can optimize performance-critical sections of code. The language’s ability to execute low-level operations, such as direct memory management, is instrumental in crafting an operating system’s kernel that balances speed and stability.
In the realm of embedded systems, C’s dominance is just as pronounced. Embedded systems are specialized computing units within larger mechanical or electrical systems, such as those found in automotive controls, medical devices, or household appliances. These systems necessitate fine-tuned control over hardware to ensure reliability and responsiveness. C’s syntactic simplicity, coupled with its powerful low-level functionality, facilitates the creation of compact, time-efficient code. This is particularly vital for embedded systems, where memory constraints and processing power are limited.
Hardware programming is yet another critical area where C excels. It transcends conventional software development by enabling interactions at the hardware level. For instance, writing firmware for microcontrollers heavily relies on C due to its ability to directly manipulate hardware registers and ports. This level of control is inexecutable with high-level programming languages. The language’s capacity to operate with minimal overhead is crucial in scenarios requiring real-time response and high reliability.
Overall, the C programming language’s low-level capabilities and robust control mechanisms make it indispensable for systems programming. Whether it’s developing an operating system, programming embedded systems, or enabling hardware interactions, C’s efficiency and versatility are unparalleled. This enduring relevance underscores the language’s fundamental role in shaping modern computing infrastructures.
Tools and Development Environments
Several tools and development environments significantly enhance the programming experience in C, ensuring efficiency and precision. Among the most crucial are compilers, integrated development environments (IDEs), and auxiliary tools like debuggers and profilers that streamline the development process.
Compilers are the backbone of C programming. They translate the human-readable code into machine code that computers can execute. The GNU Compiler Collection (GCC) is one of the most widely used compilers, known for its robustness and support for various platforms and languages. Clang, developed by the LLVM project, is another popular choice, praised for its fast compilation times and comprehensive diagnostics. Microsoft Visual C++ (MSVC) stands out for those developing on Windows, offering a seamless integration with the Visual Studio ecosystem.
Integrated Development Environments (IDEs) provide a cohesive environment that consolidates coding, compiling, and debugging into a single interface. Eclipse, a versatile and extensible IDE, supports multiple programming languages, including C, and offers a vast range of plugins that enhance its functionality. Code::Blocks, another prominent IDE, is designed specifically for C, C++, and Fortran, providing a user-friendly interface and flexibility through its plugin architecture.
Beyond compilers and IDEs, numerous tools play a critical role in the C development process. Debuggers like GDB (GNU Debugger) are essential for identifying and rectifying errors in code, offering features such as breakpoints, step execution, and backtrace. Profilers, such as Valgrind, are indispensable for performance tuning, enabling developers to analyze program execution, memory usage, and identify bottlenecks. Additionally, tools like Make and CMake facilitate the build process, automating compilation and linking to streamline project management.
In conclusion, the array of tools and development environments available for C programming—from powerful compilers to advanced debuggers and versatile IDEs—empowers developers to craft efficient, reliable, and high-performance applications. The choice of tools and environments can significantly impact the development experience, making the right selection paramount to a successful project.
Common Pitfalls and Best Practices
When working with the C programming language, both new and experienced developers should be aware of several common pitfalls and best practices to mitigate potential issues. Understanding these challenges and adopting sound strategies can significantly enhance the safety, maintainability, and efficiency of your code.
One common pitfall in C programming is the risk of memory leaks. Memory not properly managed can lead to resource exhaustion, affecting software performance over time. To avoid memory leaks, ensure that every malloc
or calloc
call has a corresponding free
statement. Utilizing tools such as Valgrind can also help identify memory leaks and other memory-related issues during development.
Buffer overflows represent another significant risk, often leading to undefined behavior or vulnerabilities. Carefully check array boundaries and use safer functions, like snprintf
instead of sprintf
, to prevent writing outside the allocated memory space. Employing static analysis tools can further help identify potential buffer overflows before they become serious issues.
Maintaining consistent coding standards is crucial for readability and collaboration. Adopt a well-defined coding style guideline, such as the Linux kernel coding style or MISRA C, to ensure that all team members write code that is uniform and easy to understand. This practice aids in code reviews and reduces the likelihood of introducing errors due to stylistic inconsistencies.
Good documentation practices are essential for long-term code maintenance. Documenting your code with clear and descriptive comments helps fellow programmers understand your logic and intentions. Writing detailed function headers that describe the purpose, inputs, outputs, and potential side effects of functions can significantly ease future maintenance and debugging tasks.
Incorporating these best practices helps C programmers avoid common pitfalls and ensures that their code is both robust and efficient. By focusing on memory management, preventing buffer overflows, adhering to coding standards, and maintaining thorough documentation, developers can produce high-quality C applications that are reliable and maintainable.
Future Trends and Conclusion
As we look to the future, the C programming language continues to hold a significant position within the evolving landscape of software development. One of the critical areas where C remains indispensable is embedded systems. Given its efficiency and control over hardware resources, C is uniquely suited for writing firmware and low-level applications that require direct hardware manipulation. As the Internet of Things (IoT) grows, the demand for proficient C programmers will remain robust.
Conversely, advancements in high-performance computing (HPC) are also sustaining the relevance of C. HPC applications necessitate highly optimized code to maximize computational performance. C, with its capacity for fine-tuning and low-level optimizations, becomes an essential tool for developers working on scientific simulations, financial modeling, and other computation-heavy tasks. While higher-level languages offer ease of use, they often lack the performance benefits that C can provide.
Moreover, developments in software practices continue to influence the landscape of C programming. Innovations such as enhanced static analysis tools, modern Integrated Development Environments (IDEs), and adaptive compilers are making C both safer and more efficient to use. Despite being over four decades old, the language is evolving to meet contemporary standards, contributing to its enduring presence in the industry.
To summarize, this exploration into the C programming language revealed its foundational characteristics and extensive applications. From its inception to its integral role in various domains, C has demonstrated remarkable staying power. Notably, its pivotal role in embedded systems and high-performance computing underscores its continued relevance. Advancements in development tools and methodologies further ensure that C remains a prime choice for developers aiming for performance and efficiency.
In the programming world, C represents both tradition and innovation. Its ability to adapt to modern requirements while maintaining its core strengths makes it a language of enduring importance and utility.