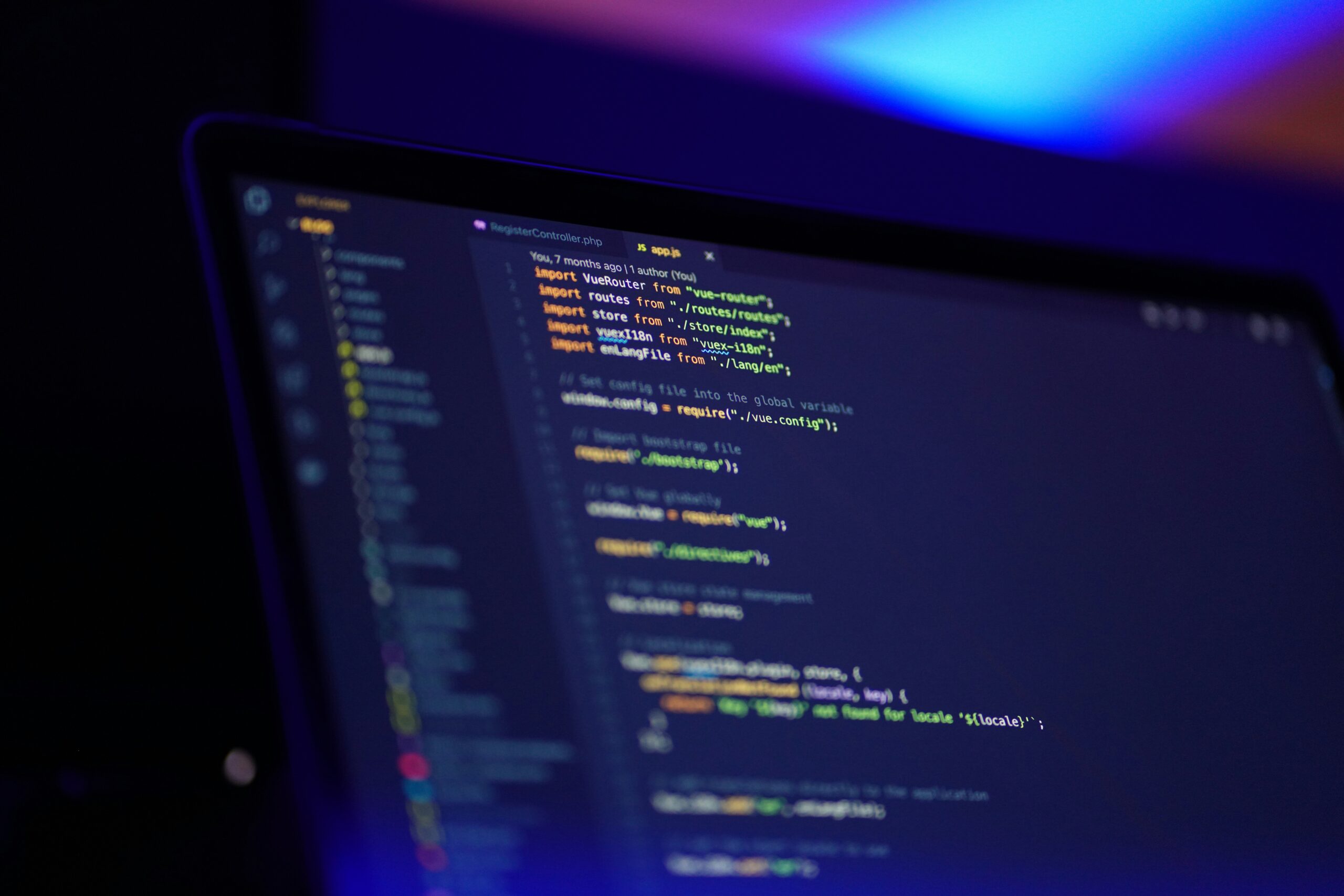
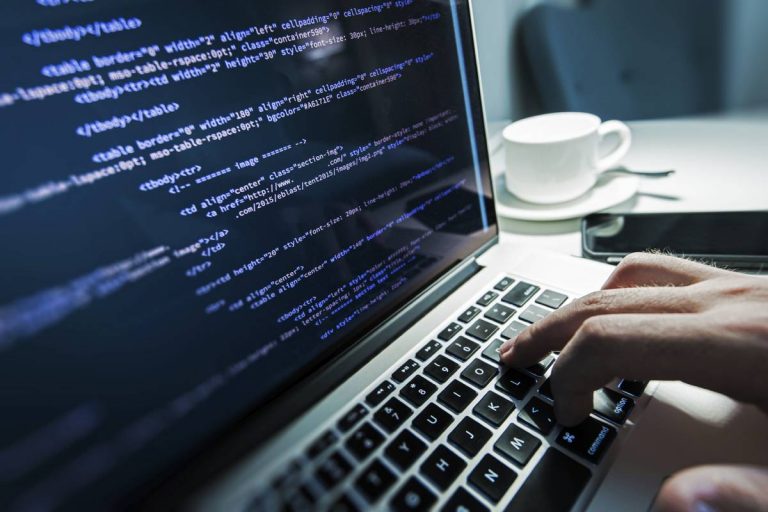
Introduction to Programming
Programming, also known as coding, involves crafting a set of instructions that a computer can execute to perform specific tasks or solve problems. At its core, programming is the process of creating precise sequences of commands that dictate how software and hardware should operate. These sequences are referred to as code.
To write effective code, programmers utilize various programming languages, which serve as the medium for communicating with computers. Each programming language has its syntax and semantics, which define the rules and meanings of its symbols and commands. Examples of popular programming languages include Python, Java, C++, and JavaScript, each designed to cater to different types of problems and applications.
The role of programming languages in this process cannot be overstated. They enable programmers to write code that computers can interpret and execute. Without programming languages, we wouldn’t have the complex software applications that power everything from personal computers to smartphones and industrial machines. Programming languages act as a bridge between human logic and machine functionality, allowing for the creation of efficient, scalable, and maintainable software.
Beyond writing code, programming also involves debugging and optimizing to ensure that the instructions work correctly and efficiently. This iterative process of writing, testing, and refining code is fundamental to developing reliable software. Understanding the logic and problem-solving techniques behind programming is essential for anyone looking to create functional and practical software solutions.
In summary, programming is the foundational skill that enables the creation and functioning of modern technology. By understanding the basic concepts of programming and leveraging various programming languages, programmers can develop innovative solutions that drive progress and efficiency in countless fields and industries.
History of Programming
The history of programming is a testament to the relentless pursuit of innovation and problem-solving. It dates back to the 19th century with Charles Babbage, who designed the first mechanical computer, the Analytical Engine. However, it was Ada Lovelace, a mathematician, who is credited with writing the first algorithm intended for a machine, making her the world’s first programmer.
The evolution of programming took a significant leap in the 1940s with the advent of electronic computers. Assembly language, one of the earliest programming languages, was developed during this period. Assembly language allowed programmers to write instructions in a format that was easier to read and understand than the raw binary code of machine language. This was a crucial milestone that significantly enhanced the efficiency of programming.
The 1950s and 1960s witnessed the creation of higher-level programming languages that further revolutionized the field. FORTRAN, developed by IBM in the 1950s, was among the first high-level languages and primarily used for scientific and engineering calculations. The 1960s brought about COBOL, designed for business data processing. These languages made programming more accessible and practical, driving advancements in various industries.
In the 1970s, the development of the C programming language marked another pivotal moment. Created by Dennis Ritchie at Bell Labs, C offered a balance of low-level efficiency and high-level readability, eventually becoming the foundation for many later languages, including C++, C#, and Java. Python, introduced in the late 1980s by Guido van Rossum, further streamlined programming with its simplicity and versatility, becoming a popular choice for web development, data analysis, artificial intelligence, and more.
Throughout the different eras, programming has not only evolved but has also significantly impacted technological advancement. The progression from machine language to high-level languages has enabled the development of more complex software, leading to breakthroughs in diverse fields such as medicine, finance, engineering, and communication. This historical journey underscores the importance of programming as a cornerstone of modern technology and innovation.
Types of Programming Languages
Programming languages can be broadly categorized based on their level of abstraction and their specific domains of application. These categories include high-level languages, low-level languages, and domain-specific languages. Each type serves a unique purpose and is tailored for particular types of tasks.
High-level programming languages are designed to be easy for humans to read and write. They abstract much of the complexity involved in managing computer hardware. Examples of high-level languages include Python and Java. Python is renowned for its simplicity and readability, making it a popular choice for beginners and for applications in web development, data science, and artificial intelligence. Java, on the other hand, is widely used in enterprise environments, particularly in server-side applications and Android app development. These high-level languages enable developers to write programs using fewer lines of code compared to their low-level counterparts, which enhances productivity and reduces the likelihood of errors.
On the other end of the spectrum are low-level programming languages, which provide little or no abstraction from a computer’s instruction set architecture. These include Assembly language and machine code. Assembly language gives programmers fine control over the hardware, as it is closely tied to the machine’s architecture. This makes it ideal for developing system software, such as operating systems and embedded systems, where efficiency and performance are paramount. Machine code, the lowest level of language, consists of binary code that the computer’s central processing unit (CPU) can execute directly. While extremely powerful, writing in machine code is highly complex and prone to errors.
Domain-specific languages (DSLs) are specialized languages designed to address particular aspects of a software problem within a specific domain. Examples include SQL for database querying, HTML for web page structuring, and MATLAB for numerical computing. DSLs are optimized for their specific tasks and provide significant efficiency by offering constructs that are specifically tailored to the domain. Consequently, they can greatly simplify development within their areas of application.
Understanding these types of programming languages and their distinct purposes helps developers choose the most appropriate tools for their specific needs, whether they are building complex software systems, managing databases, or creating web applications.
Fundamental Concepts in Programming
At its core, programming is the art of instructing a computer to perform specific tasks. To achieve this, several fundamental concepts must be understood and mastered. Among these, algorithms, data structures, control structures, syntax, and semantics are key pillars that support the creation of effective and efficient code.
Algorithms are step-by-step procedures or formulas for solving problems. They are the logical sequences that determine how a program processes data to produce the desired output. Writing a good algorithm requires precision, as it not only defines the tasks but also the order in which these tasks are executed, ensuring that the program runs smoothly.
Data structures refer to the ways in which data is organized, stored, and manipulated within a program. Common examples include arrays, linked lists, stacks, queues, and trees. The choice of an appropriate data structure is crucial because it impacts the efficiency and performance of the program. A well-selected data structure can significantly speed up data access and manipulation.
Another critical concept is control structures, which are constructs that dictate the flow of control within a program. They include conditional statements (if-else), loops (for, while), and switches, which guide decision-making processes and repetitive tasks within the code. Control structures are essential for implementing logic and ensuring that the program behaves as expected under various conditions.
Syntax refers to the set of rules that defines the combinations of symbols considered to be correctly structured programs in a programming language. Every programming language has its unique syntax, which must be followed strictly to avoid errors. Familiarity with syntax is fundamental as it ensures that the code can be compiled and executed by the computer.
Lastly, semantics involve the meaning behind the code—the logic and the intent of the instructions written by the programmer. Understanding both syntax and semantics is crucial for developing clear and purposeful programs that achieve their intended functions effectively.
Mastering these foundational concepts in programming is imperative for anyone aspiring to write robust, efficient, and scalable code. They provide a structured framework that guides the logical flow and efficient data management necessary for complex software development.
Programming Paradigms
Programming paradigms are fundamental styles of programming that provide various ways to conceptualize and solve problems. They shape the design and structure of software applications by dictating how programming tasks are approached. Four primary paradigms dominate the landscape: procedural, object-oriented, functional, and declarative programming.
Procedural programming is characterized by the step-by-step execution of instructions. It relies on procedures, also known as routines or functions, to process data. Languages like C and Pascal exemplify this paradigm. Procedural programming is particularly effective in scenarios requiring detailed task sequences and is favored for its straightforward approach. However, it often results in code that is difficult to manage and scale for larger applications, leading to challenges in maintaining and updating the codebase.
Object-oriented programming (OOP) organizes code around objects, which represent real-world entities with attributes (data) and methods (functions). Java, Python, and C++ are prominent OOP languages. This paradigm excels in scenarios involving complex systems, such as web applications and game development. Its modularity and reusability facilitate scaling and maintenance. However, OOP can introduce complexity with its abstraction and inheritance concepts, making it challenging for beginners to grasp initially.
Functional programming treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. Languages like Haskell, Scala, and Erlang are synonymous with this paradigm. Functional programming is particularly powerful in parallel processing and concurrent system architectures due to its stateless nature. Despite its advantages in promoting cleaner and more predictable code, the paradigm’s steep learning curve and the shift in mindset required can pose significant challenges.
Declarative programming focuses on the “what” rather than the “how,” specifying what the desired outcome is without dictating step-by-step instructions. SQL and HTML are common examples. This paradigm shines in database querying, configuration, and domain-specific languages due to its concise and expressive syntax. While declarative programming can significantly reduce development time, it sometimes lacks the flexibility and control provided by more imperative paradigms.
Understanding these paradigms empowers developers to choose the right approach for their specific problem, leveraging the strengths of each while mitigating their inherent challenges.
Applications of Programming
Programming has permeated virtually every sector, fundamentally redefining how processes and systems operate across various fields and industries. Among its most significant applications is software development. Programmers create applications and systems software, enabling businesses and individuals to accomplish tasks more effectively and efficiently. This extends from utility software to complex enterprise systems that underpin global business operations.
In the realm of web development, programming is essential. Developers use programming languages like HTML, CSS, and JavaScript to build and maintain websites and web applications, driving the modern digital experience. Programming ensures these platforms are not just functional but also optimized for user engagement and interaction.
Data science represents another critical area where programming plays an indispensable role. Professionals in this domain leverage languages such as Python and R to analyze massive datasets, uncovering insights that inform business decisions and strategies. This capability is pivotal for data-driven innovation in industries ranging from finance to healthcare.
Artificial intelligence (AI) and machine learning are burgeoning fields heavily reliant on programming. Here, programming languages such as Python and JavaScript are used to develop algorithms that can learn and make decisions, mimicking human intelligence. These advancements are revolutionizing industries by automating processes, enhancing accuracy, and enabling the development of smart technologies.
In robotics, programming is the backbone that enables machines to perform tasks autonomously or with minimal human intervention. Through languages like C++ and Python, robots can be programmed for precision tasks in manufacturing, healthcare, and even space exploration. Programming thus fuels advancements that enhance efficiency and capabilities in these high-tech fields.
Game development is another vibrant application of programming. Using languages such as C++ and C#, programmers design the complex algorithms that power modern video games, creating immersive and interactive experiences for users. This industry blends creativity with technical proficiency, showcasing programming as the driving force behind innovative entertainment solutions.
Ultimately, programming serves as the cornerstone of technological advancement and innovation. Its applications across these diverse fields underscore its critical role in enhancing efficiency, driving progress, and enabling new possibilities. As a result, programming stands as a key contributor to evolving industries and shaping the future of technology.
The Importance of Learning Programming
In today’s technologically advanced world, the importance of learning programming cannot be overstated. Understanding the fundamentals of programming equips individuals with a powerful toolset for problem-solving and logical thinking. These skills are not just applicable to computer science but extend to various aspects of life including decision-making and strategic planning. By learning how to code, one can break down complex problems into manageable parts, which fosters a systematic approach to solutions.
Moreover, programming skills can significantly enhance career prospects. In a rapidly digitalizing economy, there is a burgeoning demand for professionals who can write code. Industries as diverse as healthcare, finance, and entertainment are increasingly reliant on software to improve efficiency and deliver innovative solutions. Consequently, individuals with programming expertise often find themselves with a competitive edge in the job market. Whether aspiring to be a software developer, data analyst, or cybersecurity expert, coding knowledge opens up a myriad of career opportunities.
Additionally, learning how to program has become increasingly accessible, thanks to numerous online courses, boot camps, and community resources. This accessibility ensures that virtually anyone, regardless of their educational background, can acquire these valuable skills. Furthermore, many educational institutions have integrated programming into their curriculums, underscoring its importance in a well-rounded education.
The significance of programming extends beyond professional and academic realms. In our everyday lives, we interact with technology in ways that are deeply influenced by coding—from the apps on our smartphones to the websites we browse. Understanding the basics of programming allows individuals to better navigate this digital landscape, enhancing their overall digital literacy.
In essence, the ability to program has become a vital skill in the 21st century. As technology continues to evolve, the relevance of programming grows concurrently, making it a critical component of modern education and professional development.
Future of Programming
The future of programming is poised for significant transformations, driven by emerging trends and technological advancements. One central development is the evolution of programming languages. As the landscape of technology rapidly changes, new languages are being developed to address specific needs, improve efficiency, and enhance security. Languages like Rust and Kotlin, for instance, are gaining popularity due to their robust security features and performance capabilities. Meanwhile, existing languages such as Python and JavaScript continue to evolve, incorporating new features that make them more versatile and powerful for modern applications.
Another realm revolutionizing programming is artificial intelligence (AI). AI is increasingly playing a pivotal role in software development, streamlining processes and enabling the creation of more sophisticated applications. Automated code generation, bug detection, and optimization are just a few examples of how AI is making programming more efficient. AI-driven development environments are also emerging, offering advanced features like predictive analytics and intelligent code suggestions, which significantly reduce development time and improve code quality.
The potential future applications of programming are vast, and they promise to transform various industries. In healthcare, for example, programming advancements are enabling the development of personalized medicine and advanced diagnostic tools. In finance, programming is central to the creation of secure blockchain technologies and advanced financial models. The automotive industry is leveraging programming for the development of autonomous vehicles and sophisticated traffic management systems. Additionally, the Internet of Things (IoT) is expanding the horizon for programming, as interconnected devices require efficient and scalable software solutions.
As we look ahead, it becomes evident that the field of programming will continue to evolve, fostering innovation across multiple sectors. The integration of AI, the emergence of new languages, and the rapid adoption of IoT are just a few indicators of how programming is poised to shape the future. As such, staying abreast of these trends and advancements is crucial for professionals in the field, ensuring they remain at the forefront of technological progress.